Relative deformation on path - Example
Options
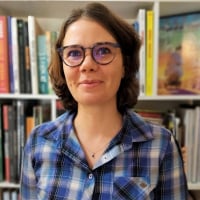
Pernelle Marone-Hitz
Member, Moderator, Employee Posts: 831
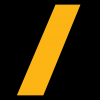
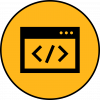
User creates manually a directional or total deformation plot on a path:
The result curve is as follows:
They would like to extract the plot data values, create a linear interpolation between the first and last value and get the relative displacement as: relativeDef = extractedDef - linearRegressionDef
Tagged:
0
Answers
-
Below script will do just that :
import wbjn import units import os def GetLinearRegression(ps,pv): x1=ps[0] x2=ps[-1] y1=pv[0] y2=pv[-1] a=(y2-y1)/(x2-x1) b=y1-a*x1 return a,b def GetPathCurvAbscissa(px,py,pz): import math ps=[] # Retrieve curvilinear abscissa. Paths are straight lines, # so retrieve length from end points length=sqrt((px[-1]-px[0])**2+(py[-1]-py[0])**2+(pz[-1]-pz[0])**2) # now get abscissa for i in range(len(px)): l=sqrt((px[i]-px[0])**2+(py[i]-py[0])**2+(pz[i]-pz[0])**2) ps.append(l) return ps,length # Retrieve all deformation results all_res=ExtAPI.DataModel.GetObjectsByType(DataModelObjectCategory.DeformationResult) path_res=[] # Check if results are scoped on a path for res in all_res: if res.ScopingMethod==Ansys.Mechanical.DataModel.Enums.GeometryDefineByType.Path: if res.Suppressed==False: path_res.append(res) if len(path_res)>0: # Results scoped on path have been found # Retrieve user_files folder cmd = 'returnValue(GetUserFilesDirectory())' user_dir = wbjn.ExecuteCommand(ExtAPI,cmd) # Write data to 'relative_path_data.csv' in project's user_files folder f=open(os.path.join(user_dir,'relative_path_data.csv'),'w') # Loop over results to find deformation results scoped on paths for res in path_res: pdata=res.PlotData.Values # Retrieve results unit and compute scale_factor to export in project units currentLengthUnit=ExtAPI.DataModel.CurrentUnitFromQuantityName('Length') scale_factor=units.ConvertUnit(1.,pdata[3].Unit,currentLengthUnit) # Split in X, Y, Z and values pdata_X=[] pdata_Y=[] pdata_Z=[] pdata_Values=[] for i in range(pdata[3].Count): pdata_X.append(pdata[0][i]*scale_factor) pdata_Y.append(pdata[1][i]*scale_factor) pdata_Z.append(pdata[2][i]*scale_factor) pdata_Values.append(pdata[3][i]*scale_factor) # Compute curvilinear abscissa pdata_S,length=GetPathCurvAbscissa(pdata_X,pdata_Y,pdata_Z) # linear regression on path values a,b=GetLinearRegression(pdata_S,pdata_Values) # Write data to csv file with ',' as separator f.write('{0},\n'.format(res.Name)) # Header f.write('{0} in {5},{1} in {5},{2} in {5},{3} in {5},{4} in {5}\n'.format('X Coord','Y Coord','Z Coord','Abscissa on path','Deformation',currentLengthUnit)) # Values for i in range(len(pdata_Values)): # this is the deformation value minus the linear regression value relative_value=pdata_Values[i]-(a*pdata_S[i]+b) f.write('{0},{1},{2},{3},{4}\n'.format(pdata_X[i],pdata_Y[i],pdata_Z[i],pdata_S[i],relative_value)) f.write('\n') f.close() else: # No results scoped on path, issue warning message ExtAPI.Application.Messages.Add(Ansys.Mechanical.Application.Message('No deformation results on path available.', MessageSeverityType.Warning))
4