Import Heat Flow tabular load using Python script
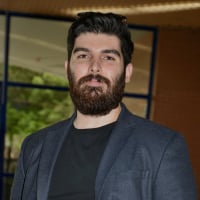
Nikos Kallitsis
Member, Employee Posts: 38
✭✭✭✭
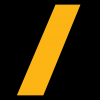
I have a Heat Flow table in .csv format, with the timesteps in the first column and the load values in the second column.
How can I import this load and apply it to a Face in Mechanical using Python?
Tagged:
0
Best Answer
-
You can import a Heat Flow load with Python using the code below.
- It's necessary to have defined the faces you want to apply the load to in a Named Selection, e.g., SelectedFaces.
- The first row of the data is assumed to be the headers, and therefore it's skipped.
import os import csv filePath = os.path.join('YourDrive', os.sep, 'File', 'Path') #Create path YourDrive:\File\Path fileName = os.path.join(filePath, 'YourFile.csv') #Create path YourDrive:\File\Path\YourFile.csv os.chdir(filePath) #Change directory timesteps = [] #Create empty list to store timestep values loads = [] #Create empty list to store load values with open(fileName, 'r') as csvFile: csvRead = csv.reader(csvFile, delimiter=',') next(csvRead) #Skip first line (assumed headers) for row in csvRead: timesteps.append(row[0]) #Populate list with first column's values loads.append(row[1]) #Populate list with second column's values #Configure lists timeUnit = '[sec]' #Timestep unit loadUnit = '[W]' #Load unit timesteps = [str(time) + timeUnit for time in timesteps] #Convert values to strings and append unit loads = [str(load) + loadUnit for load in loads] #Convert values to strings and append unit #Create load analysis = Model.Analyses[0] #Access 1st analysis system heatFlow = analysis.AddHeatFlow() #Add a Heat Flow load to analysis loadFace = DataModel.GetObjectsByName('SelectedFaces')[0] #Get Named Selection with load face heatFlow.Location = loadFace #Scope load to Named Selection heatFlow.Magnitude.Inputs[0].DiscreteValues = [Quantity(time) for time in timesteps] heatFlow.Magnitude.Output.DiscreteValues = [Quantity(load) for load in loads]
3
Answers
-
Hi, I tried your code, and it works pretty well. Unfortunately, the step controls in the analysis settings is not updated in my model. Could you help me how to fix it?
0