Python code errors
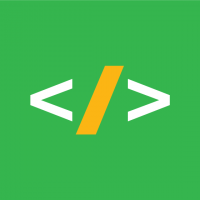
I am trying to run two codes, attached, and receiving the following error messages:
at line 11: if app.ProjectName != project_name:
The first code to generate the antenna:
import os import win32com.client # Create an instance of HFSS application app = win32com.client.Dispatch("AnsoftHfss.HfssScriptInterface") # Initialize HFSS project and design project_name = "Dipole_Antenna" design_name = "Dipole_Design" if app.ProjectName != project_name: app.NewProject(project_name) if app.ActiveDesignName != design_name: app.InsertDesign("HFSS", design_name) app.SetActiveDesign(design_name) design = app.GetActiveDesign() # Create a new HFSS design setup for the simulation setup = design.InsertSetup("HfssDrivenModal", "Setup1", "Frequency", ["GHz", False, 1], ["Max Delta S:=", 0.01, "Use Maximum Delta S:=", False, "Basis Order:=", 1, "Do Lambda Refinement:=", True]) # Create the dipole antenna geometry wire_radius = 2e-3 # 2 mm in meters arm_height = 0.5 # Arm height arm1 = design.DrawCylinder( [ "NAME:CylinderParameters", "XCenter:=", "0mm", "YCenter:=", "0mm", "ZCenter:=", "-0.25mm", "Radius:=", f"{wire_radius}m", "Height:=", f"{arm_height}mm", "WhichAxis:=", "Z", "NumSides:=", "0" ], [ "NAME:Attributes", "Name:=", "Arm1", "Flags:=", "", "Color:=", "(132 0 255)", "Transparency:=", 0.5, "PartCoordinateSystem:=", "Global", "UDMId:=", "", "MaterialValue:=", "\"vacuum\"", "SurfaceMaterialValue:=", "\"\"", "SolveInside:=", True, "IsMaterialEditable:=", True, "UseMaterialAppearance:=", False, "IsLightweight:=", False ]) arm2 = design.DrawCylinder( [ "NAME:CylinderParameters", "XCenter:=", "0mm", "YCenter:=", "0mm", "ZCenter:=", "0.25mm", "Radius:=", f"{wire_radius}m", "Height:=", f"{arm_height}mm", "WhichAxis:=", "Z", "NumSides:=", "0" ], [ "NAME:Attributes", "Name:=", "Arm2", "Flags:=", "", "Color:=", "(132 0 255)", "Transparency:=", 0.5, "PartCoordinateSystem:=", "Global", "UDMId:=", "", "MaterialValue:=", "\"vacuum\"", "SurfaceMaterialValue:=", "\"\"", "SolveInside:=", True, "IsMaterialEditable:=", True, "UseMaterialAppearance:=", False, "IsLightweight:=", False ]) # Create a vacuum region vacuum = design.CreateRegion( ["NAME:Vacuum", "RegionPrimitive:=", "Box", "XStart:=", "-100mm", "YStart:=", "-100mm", "ZStart:=", "-100mm", "XEnd:=", "100mm", "YEnd:=", "100mm", "ZEnd:=", "100mm"]) # Assign materials to objects design.AssignMaterial( ["NAME:Arm1", "Objects:=", [f"{arm1.GetPath()}"], "Material:=", "vacuum"]) design.AssignMaterial( ["NAME:Arm2", "Objects:=", [f"{arm2.GetPath()}"], "Material:=", "vacuum"]) # Add a driven modal port port_location = (-arm_height / 2) * 1e-3 # Convert to meters port_name = "Port1" port = design.CreateWavePort( [ "NAME:" + port_name, "Objects:=", [f"{arm1.GetPath()}"], "DoDeembed:=", False, "DoAdapt:=", False, "Port Name:=", port_name, "Y0 Position:=", f"{port_location}m", "Y0 Delta:=", "0.001m", "Z0 Position:=", "0.001m", "Z0 Delta:=", "0.001m" ]) # Create an excitation setup excitation_name = "Excitation" setup.EditSources( [ "NAME:" + excitation_name, "Voltage:=", "1V", "Do Pattern:=", False, "Phase:=", "0deg" ]) # Save the project project_dir = os.path.join(os.getcwd(), "HFSS_Project") if not os.path.exists(project_dir): os.makedirs(project_dir) project_path = os.path.join(project_dir, f"{project_name}.aedt") app.SaveProjectAs(project_path) print(f"Project saved at: {project_path}") The second code to install the antenna on a simple vehicle model import os import win32com.client # Create an instance of HFSS application app = win32com.client.Dispatch("AnsoftHfss.HfssScriptInterface") # Initialize HFSS project and design project_name = "Vehicle_Antenna" design_name = "Vehicle_Design" if app.ProjectName != project_name: app.NewProject(project_name) if app.ActiveDesignName != design_name: app.InsertDesign("HFSS", design_name) app.SetActiveDesign(design_name) design = app.GetActiveDesign() # Create a new HFSS design setup for the simulation setup = design.InsertSetup("HfssDrivenModal", "Setup1", "Frequency", ["GHz", False, 1], ["Max Delta S:=", 0.01, "Use Maximum Delta S:=", False, "Basis Order:=", 1, "Do Lambda Refinement:=", True]) # Define vehicle dimensions vehicle_length = 4000 # mm vehicle_width = 1800 # mm vehicle_height = 1500 # mm # Create the vehicle geometry vehicle = design.DrawBox( [ "NAME:BoxParameters", "XPosition:=", "-{0}mm".format(vehicle_length / 2), "YPosition:=", "-{0}mm".format(vehicle_width / 2), "ZPosition:=", "0mm", "XSize:=", "{0}mm".format(vehicle_length), "YSize:=", "{0}mm".format(vehicle_width), "ZSize:=", "{0}mm".format(vehicle_height) ], [ "NAME:Attributes", "Name:=", "Vehicle", "Flags:=", "", "Color:=", "(255 132 132)", "Transparency:=", 0.5, "PartCoordinateSystem:=", "Global", "UDMId:=", "", "MaterialValue:=", "\"pec\"", "SurfaceMaterialValue:=", "\"\"", "SolveInside:=", True, "IsMaterialEditable:=", True, "UseMaterialAppearance:=", False, "IsLightweight:=", False ]) # Create the dipole antenna geometry as before # ... # Position the dipole antenna at the center of the vehicle's top surface antenna_offset = vehicle_height / 2 # Move the antenna to the desired position arm1.Move( [ "NAME:All", "Translate:=", ["0mm", "0mm", f"{antenna_offset}mm"] ]) # Add a driven modal port # ...
Create an excitation setup
...
Save the project
project_dir = os.path.join(os.getcwd(), "HFSS_Project")
if not os.path.exists(project_dir):
os.makedirs(project_dir)
project_path = os.path.join(project_dir, f"{project_name}.aedt")
app.SaveProjectAs(project_path)
print(f"Project saved at: {project_path}")
Answers
-
Appreciate your help to resolve the errors. Thank you
0 -
@Hussain Al-Rizzo a couple of things that will help get your question looked at by someone who can answer:
1) I have formatted your code as code, although it is too long for a post, and I am not sure it is right. You should try and break it up into just the parts that are giving you issues and use more generic examples if possible so that it can be parsed and answered more quickly.
2) If you can you should go in and edit the post and tag it with the application(s) and tool(s) you are using as that will make sure that the right SME teams see it.Once you have done that, we can point folks at your question and get it addressed! Thanks,
Chris0