How can I pull Peak Irradiance or Total Hits from the Detector Viewer analysis in the API?
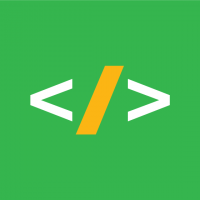
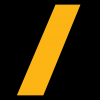
Within the API, we are able to quickly pull Peak Irradiance, Total Power, and Total Hits by using the property GetDetectorData
as described in the forum post 'Methods for extracting detector data through the API.' However, this property will not take into account an applied ZRD file. We can apply a ZRD file to a Detector Viewer window, so is there a way to extract the same information from there?
Best Answer
-
I will write my answer in Matlab, but – ignoring minor syntax differences – the process should be very similar across languages!
When we work with the Detector Viewer analysis window, we can access the results interface with the following:
det = TheSystem.Analyses.New_Analysis(ZOSAPI.Analysis.AnalysisIDM.DetectorViewer); det.ApplyAndWaitForCompletion; detResults = det.GetResults();
This allows us to extract any text data from the Detector Viewer analysis window. This will include most items in the Text tab, and most items in the Beam Info tab of the Detector Viewer.
Within the
detResults
object, we will find a few other properties. In the case of a detector with a non-Cross Section output, we will have the following:The populated properties represent the following:
- MetaData: This holds lens data information, such as the title and date:
- HeaderData: This holds much of the information that comes before the raw pixel-by-pixel output. Note: the rest of the information (Data Type, detector tilt, etc.) can be extracted from the Detector Viewer Settings interface, or the NCE Row interface
- DataGrid: This holds nxn matrix data which corresponds to the pixel-by-pixel output. This can be converted into an image which matches the view in the Graph tab, as discussed in the forum post 'How do I output the image of an analysis in ZOS-API?'
- DataSeries: This holds the information from the Beam Info tab.
To extract 'Peak Irradiance' from the Detector Viewer, we want to use the HeaderData property. We can access this by using a dot index. This dot indexer will combine the object I'm currently in (
detResults
) with the title of the property I want to access (HeaderData
):headerData = detResults.HeaderData
Within
HeaderData
, we are given only a String vector titled Lines:After investigation of this property, we see that this string vector has a 'Length' of '5'. That means there are 5 lines from the text output which we can access. For the Detector Viewer I am using, the line numbers will correspond to the following:
In this case, Line 5 is blank. To extract this data into the API, we use dot indexing again:
peakIrradiance = string(headerData.Lines(3));
The output of
peakIrradiance
will then be the entire line 'Peak Irradiance : 6.7027E+05 Watts/cm^2'. We can use program-specific operations to truncate this and convert it to a double value. For example, in Matlab, I can useextractBefore
,extractAfter
, andstr2double
to output the numeric value alone. Here is what that would look like:peakIrradiance = str2double(extractBefore(extractAfter(string(headerData.Lines(3)), ': '), ' Watts'));
This method can be carried across all of the
HeaderData
values within the Detector Viewer analysis. Here is a full example, run after a ray trace:% Open Detector det = TheSystem.Analyses.New_Analysis(ZOSAPI.Analysis.AnalysisIDM.DetectorViewer); det.ApplyAndWaitForCompletion; detResults = det.GetResults(); headerData = detResults.HeaderData; totalHits = str2double(extractAfter(string(headerData.Lines(2)), 'Total Hits = ')); peakIrradiance = str2double(extractBefore(extractAfter(string(headerData.Lines(3)), ': '), ' Watts')); totalPower = str2double(extractBefore(extractAfter(string(headerData.Lines(4)), ': '), ' Watts')
0