How can I export a GLB / GLTF file format with PyDPF?
Options
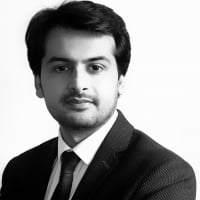
Ayush Kumar
Member, Moderator, Employee Posts: 419
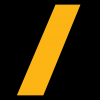
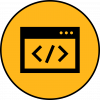
Comments
-
You will need to install any Python lib. to convert GLTF to GLB format because PyVista only exports GLTF. An example would be pip installing
pygltflib
import os from ansys.dpf import core as dpf from ansys.dpf.core.plotter import DpfPlotter from pygltflib.utils import gltf2glb out_path = r"\FOLDER\PATH\TO\RESULT\FILE" rst_path = os.path.join(out_path, "file.rst") model = dpf.Model(rst_path) mesh = model.metadata.meshed_region print(mesh) displacement = model.results.displacement() plotter = DpfPlotter() plotter.add_field(displacement.outputs.fields_container()[0]) gltf_path = os.path.join(out_path, r"test_export.gltf") gltf = plotter._internal_plotter._plotter.export_gltf(gltf_path) glb_path = os.path.join(out_path, r"test_export.glb") """Convert GLTF to GLB File""" gltf2glb(gltf_path, glb_path) """ Export just legend as an image with white Background Can be used as a background in a 3D viewer where the GLB is imported. """ plotter = DpfPlotter() plotter.add_field(disp_f, opacity=0.0) path_to_legend = os.path.join(out_path, "legend.png") plotter.show_figure(screenshot=path_to_legend)
0 -
There is a custom operator created as an example for this
once loaded the usage is straightforward
new_operator = dpf.Operator("gltf_export") import os model = dpf.Model(dpf.upload_file_in_tmp_folder(examples.find_static_rst())) mesh = model.metadata.meshed_region skin_mesh = dpf.operators.mesh.tri_mesh_skin(mesh=mesh) displacement = model.results.displacement() displacement.inputs.mesh_scoping(skin_mesh) displacement.inputs.mesh(skin_mesh) new_operator.inputs.path(os.path.join(tmp, "out")) new_operator.inputs.mesh(skin_mesh) new_operator.inputs.field(displacement.outputs.fields_container()[0]) new_operator.run() print("operator ran successfully") dpf.download_file(os.path.join(tmp, "out.glb"), os.path.join(os.getcwd(), "out.glb"))
1 -
Just wanted to point out that the DPF's
gltf_export
also usespygltflib
behind the scenes.1