How do I extract Mode Shapes with Result file on remote machine using PyDPF?
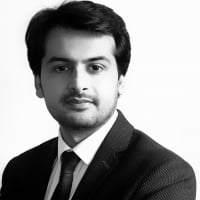
Ayush Kumar
Member, Moderator, Employee Posts: 468
✭✭✭✭
Comments
-
Replace the remote DPF server's IP and port in line 13 and RST file path on the remote machine in line 14.
import time from ansys.dpf import core as dpf from ansys.dpf.core.plotter import DpfPlotter from ansys.dpf.core import animation from ansys.dpf.core import examples ############################################################################### # Retrieve mode shapes # ~~~~~~~~~~~~~~~~~~~~ t1 = time.time() server = dpf.connect_to_server(ip="<IP>", port=<port>) rst_path = r"\Path\to\file.rst" # Load the result file as a model model = dpf.Model(rst_path) n_modes = model.metadata.time_freq_support.n_sets freqs = model.metadata.time_freq_support.time_frequencies.data t2 = time.time() print("Time taken to calculate freqs - %s seconds" % round((t2 - t1), 2)) time_scoping = dpf.Scoping() time_scoping.ids = range(1, n_modes + 1) print(model.metadata.meshed_region) # Skin Mesh skin_mesh = dpf.operators.mesh.skin(mesh=model.metadata.meshed_region) skin_op = skin_mesh.outputs.mesh.get_data() print(skin_op) t11 = time.time() # Extract the displacement results which define mode shapes disp = model.results.displacement(mesh_scoping=skin_mesh.outputs.nodes_mesh_scoping, time_scoping=time_scoping).outputs.fields_container.get_data() t21 = time.time() print("Time taken to extract displacements - %s seconds" % round((t21 - t11), 2)) ############################################################################### # Plot mode shapes # ~~~~~~~~~~~~~~~~ # Get the frequency scoping (available frequency IDs for disp) freq_scoping = disp.get_time_scoping() # Get the frequency support (all available frequencies in the model) freq_support = disp.time_freq_support # Get the unit from the time_freq_support unit = freq_support.time_frequencies.unit # For each ID in the scoping for index, freq_set in enumerate(freq_scoping): t10 = time.time() # Get the associated frequency in the time_freq_support freq = freq_support.get_frequency(cumulative_index=freq_set - 1) # Get the associated mode shape as a displacement field disp_mode = disp.get_field_by_time_complex_ids(freq_set, 0) data = disp_mode.data t20 = time.time() print("Time taken to extract Mode-%s is %s seconds" % (freq_set, round((t20 - t10), 2)))
1