Transfer convection coefficient and ambient temperature data from csv to Mechanical via PyMechanical
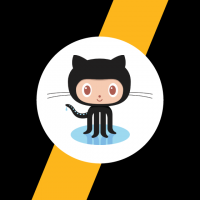
I have data in csv and want to replace the convection coeff and ambient temperatures of convection boundary conditions in my mechanical model with the values in csv. How can I do this in PyMechanical?
Comments
-
You can use PyMechanical to,
- Open an existing mechdat file (one can export mechdat from Mechanical --> File --> Export)
- Read in inputs (say from csv) using csv Module
- Replace the boundary condition inputs from the inputs from csv
- Save this modified mechanical model as a new mechdat
Attached is a simple PyMechanical (Remote Session) Script to do the same in 2023R2.
import os import ansys.mechanical.core as pymechanical from ansys.mechanical.core import find_mechanical wb_exe = find_mechanical(232)[0] UserDir = r"D:\Cases\26832" commandFileName = r"commandFile.py" commandFilePath = os.path.join(UserDir,commandFileName) mechanical = pymechanical.launch_mechanical(exec_file=wb_exe, batch=False) print(mechanical) mechanical.run_python_script_from_file(commandFilePath)
The above script needs the file "commandFile.py", which has the below script. This script reads in the csv file (whose data is pasted at the end) which has Convection Object name, Film Coefficient, Film Coeff unit, Ambient Temperature and Ambient Temperature unit data for multiple Convection boundary conditions. Then based on Convection name in the csv it finds the corresponding convection object in the analysis and replaces the inputs (Film Coefficient, Ambient Temperature - constant values i.e. not tabular) for this convection object. Then it exports the modified mechdat (to verify).
import os import csv filenameMechDb = r"MECHinput.mechdat" filepath = r"D:\Cases\26832" filepathMechDb = os.path.join(filepath,filenameMechDb) filenameCSV = r"Convection_Data.csv" filepathCSV = os.path.join(filepath,filenameCSV) fileNew = r"MECHinput_modified.mechdat" fileNewPath = os.path.join(filepath,fileNew) ExtAPI.DataModel.Project.Open(filepathMechDb) convData = [] with open(filepathCSV, 'r') as csvfile: csv_reader = csv.reader(csvfile) for row in csv_reader: print(row) convName = row[0] convFCVal = row[1] convFCUnit = row[2] convATVal = row[3] convATUnit = row[4] convData.append([convName, convFCVal, convFCUnit, convATVal, convATUnit]) for i in range(len(convData)): ConvObj = DataModel.GetObjectsByName(convData[i][0])[0] lastIndexConv = ConvObj.FilmCoefficient.Output.DiscreteValueCount-1 lastIndexAT = ConvObj.AmbientTemperature.Output.DiscreteValueCount-1 ConvObj.FilmCoefficient.Output.SetDiscreteValue(lastIndexConv, Quantity(float(convData[i][1]), convData[i][2])) ConvObj.AmbientTemperature.Output.SetDiscreteValue(lastIndexConv, Quantity(float(convData[i][3]), convData[i][4])) ExtAPI.DataModel.Project.Save(fileNewPath)
csv Data:
Convection_out1, 0.0003, W mm^-1 mm^-1 C^-1, 726.85, C,
Convection_in1, 0.0003, W mm^-1 mm^-1 C^-1, 14.85, C,
Convection_in2, 0.000301, W mm^-1 mm^-1 C^-1, 15.85, C,
Convection_in3, 0.000302, W mm^-1 mm^-1 C^-1, 16.85, C,
Convection_in4, 0.000303, W mm^-1 mm^-1 C^-1, 26.85, C,
Convection_in5, 0.000304, W mm^-1 mm^-1 C^-1, 27.85, C,
Convection_in6, 0.000305, W mm^-1 mm^-1 C^-1, 28.85, C,
Convection_hole1, 0.0002, W mm^-1 mm^-1 C^-1, 14.85, C,
Convection_hole2, 0.000201, W mm^-1 mm^-1 C^-1, 15.85, C,
Convection_hole3, 0.000202, W mm^-1 mm^-1 C^-1, 16.85, C,
Convection_hole4, 0.000204, W mm^-1 mm^-1 C^-1, 26.85, C,
Convection_hole5, 0.000205, W mm^-1 mm^-1 C^-1, 27.85, C,
Convection_hole1 2, 0.000206, W mm^-1 mm^-1 C^-1, 28.85, C,
Convection_hole2 2, 0.000207, W mm^-1 mm^-1 C^-1, 29.85, C,
Convection_hole3 2, 0.000208, W mm^-1 mm^-1 C^-1, 30.85, C,
Convection_hole4 2, 0.000209, W mm^-1 mm^-1 C^-1, 31.85, C,
Convection_hole5 2, 0.00038, W mm^-1 mm^-1 C^-1, 32.85, C,0 -
The same can also be done via Embedded option as shown below.
import os import logging import csv from ansys.mechanical.core import App from ansys.mechanical.core.embedding.logger import (Configuration,Logger) Configuration.configure(level=logging.WARNING,to_stdout=True) app = App(version=232) print(app) Logger.error("message") # Extract the global API entry points (available from built-in Mechanical scripting) from ansys.mechanical.core import global_variables # Merge them into your Python global variables globals().update(global_variables(app)) filenameMechDb = r"MECHinput.mechdat" filepath = r"D:\Cases\26832" filepathMechDb = os.path.join(filepath,filenameMechDb) filenameCSV = r"Convection_Data.csv" filepathCSV = os.path.join(filepath,filenameCSV) fileNew = r"MECHinput_modified2.mechdat" fileNewPath = os.path.join(filepath,fileNew) ExtAPI.DataModel.Project.Open(filepathMechDb) convData = [] with open(filepathCSV, 'r') as csvfile: csv_reader = csv.reader(csvfile) for row in csv_reader: print(row) convName = row[0] convFCVal = row[1] convFCUnit = row[2] convATVal = row[3] convATUnit = row[4] convData.append([convName, convFCVal, convFCUnit, convATVal, convATUnit]) for i in range(len(convData)): ConvObj = DataModel.GetObjectsByName(convData[i][0])[0] lastIndexConv = ConvObj.FilmCoefficient.Output.DiscreteValueCount-1 lastIndexAT = ConvObj.AmbientTemperature.Output.DiscreteValueCount-1 ConvObj.FilmCoefficient.Output.SetDiscreteValue(lastIndexConv, Quantity(float(convData[i][1]), convData[i][2])) ConvObj.AmbientTemperature.Output.SetDiscreteValue(lastIndexConv, Quantity(float(convData[i][3]), convData[i][4])) ExtAPI.DataModel.Project.Save(fileNewPath) app.close()
0