Python Result: select time step, named selection, and direction
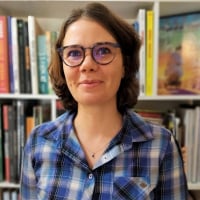
Pernelle Marone-Hitz
Member, Moderator, Employee Posts: 871
✭✭✭✭
in Structures
Do you have an example of Python Result where the user can select:
- the display time
- a named selection on which to calculate and plot the result
- the direction of the deformation to be evaluated and plotted
?
Tagged:
0
Answers
-
Here's an example.
The Property Provider is edited to define:three user inputs:
- the direction of the deformation (drop-down menu)
- the display time (provide a float or int)
- a named selection (drop-down menu)
two parametrizable outputs:
- the mean value of the plot
- the max value of the plot
def reload_props(): this.PropertyProvider = None provider = Provider() group = provider.AddGroup("My Properties") # Select component for plotting directional deformation direction_prop = group.AddProperty("Directional deformation", Control.Options) direction_prop.Options = {0: 'UX', 1: 'UY', 2: 'UZ'} # Define time for postprocessing time_prop = group.AddProperty("Display Time", Control.Double) # DropDown to select a named selection selection_prop = group.AddProperty("Named selection", Control.Options) # Retrieve all named selections and fill the dropdown with the list of named selections all_named_sel=DataModel.GetObjectsByType(DataModelObjectCategory.NamedSelection) ns_list={} idx=1 for ns in all_named_sel: ns_list[idx]=ns.Name idx+=1 selection_prop.Options = ns_list # Second group for some results group2 = provider.AddGroup("My Results") # Mean value mean_prop = group2.AddProperty("Mean value", Control.Double) mean_prop.ParameterType = ParameterType.Output mean_prop.CanParameterize = True # Maximum value max_prop = group2.AddProperty("Maximum value", Control.Double) max_prop.ParameterType = ParameterType.Output max_prop.CanParameterize = True # Connects the provider instance back to the object by setting the PropertyProvider member on this, 'this' being the # current instance of the Python Code object. this.PropertyProvider = provider
As for the script itself, it should be as follows:
def define_dpf_workflow(analysis): # Import library and reference DataSources import mech_dpf import Ans.DataProcessing as dpf mech_dpf.setExtAPI(ExtAPI) dataSources = dpf.DataSources(analysis.ResultFileName) #Retrieve properties direction = this.GetCustomPropertyByPath("My Properties/Directional deformation").ValueString time = float(this.GetCustomPropertyByPath("My Properties/Display Time").Value) selection_name = this.GetCustomPropertyByPath("My Properties/Named selection").ValueString # Get selection corresponding to user choice if selection_name!='': scoping_op=dpf.operators.scoping.on_named_selection(data_sources=dataSources,named_selection_name=selection_name.ToUpper()) scoping=scoping_op.outputs.getmesh_scoping() # Get deformation with scoping choices defined by the user u = dpf.operators.result.displacement() u.inputs.data_sources.Connect(dataSources) u.inputs.mesh_scoping.Connect(scoping) u.inputs.time_scoping.Connect(time) # Get component direction_map = {'UX': 0, 'UY': 1, 'UZ': 2} comp_int = direction_map.get(direction.upper(), None) component = dpf.operators.logic.component_selector() component.inputs.field.Connect(u) component.inputs.component_number.Connect(comp_int) component.inputs.default_value.Connect(1) component.outputs.field.GetData() # Set output results max=dpf.operators.min_max.min_max(component) maxval=max.outputs.getfield_max().Data[0] cpmdata=component.outputs.field.GetData().Data mean=sum(list(cpmdata))/len(cpmdata) this.GetCustomPropertyByPath("My Results/Mean value").Value=mean this.GetCustomPropertyByPath("My Results/Maximum value").Value=maxval # Set plot dpf_workflow = dpf.Workflow() dpf_workflow.Add(component) dpf_workflow.SetOutputContour(component) dpf_workflow.Record('wf_id', False) this.WorkflowId = dpf_workflow.GetRecordedId()
0