How to plot contact reaction FY force against frequency in a harmonic analysis using PyMAPDL?
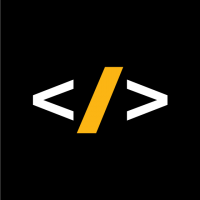
Javier Vique
Member, Employee Posts: 86
✭✭✭✭
in Structures
Usually, when we want to plot variables against time/frequency, we make use of POST26. However, POST26 does not have FSUM capability.
Tagged:
0
Answers
-
The script below gets the contact reaction forces in Y direction for elemental contact types 4 and 6 and plots them for the first type against frequency:
import os from ansys.mapdl.core import launch_mapdl import matplotlib.pyplot as plt Root_Folder = r'here_your_path' PyMAPDL_Folder = os.path.join(Root_Folder,'PyMAPDL') rst_file = os.path.join(PyMAPDL_Folder,'file') mapdl = launch_mapdl(license_server_check=False, run_location=PyMAPDL_Folder, port=50046) mapdl.post1() mapdl.file(fname = rst_file, ext ='rst') result = mapdl.result freqs = result.time_values[1::2] fy_real_all = [] fy_imag_all = [] conta174_etypes = [4,6] for j in conta174_etypes: fy_real = [] fy_imag = [] mapdl.set(lstep = 0) mapdl.allsel() mapdl.esel('S', 'TYPE', '', j) mapdl.nsle() mapdl.esln() mapdl.esel('U', 'TYPE', '', j) for i in freqs: mapdl.set(lstep='', sbstep='', fact='', kimg=0, time=i, angle='', nset='', order='') fsum = mapdl.fsum() fy_real.append(-float(fsum.split('\n')[2].split('=')[1].strip())) mapdl.set(lstep='', sbstep='', fact='', kimg=1, time=i, angle='', nset='', order='') fsum = mapdl.fsum() fy_imag.append(-float(fsum.split('\n')[2].split('=')[1].strip())) fy_real_all.append(fy_real) fy_imag_all.append(fy_imag) mapdl.exit() plt.plot(freqs, fy_real_all[0]) plt.title('Real FY vs freq of conta type %d' % conta174_etypes[0])
0 -
An alternative way would be to use DPF operators for element nodal forces and the accumulate over fields container operator to do an effective "FSUM" operation. This would be with pyDPF and should be more efficient especially with high freq. counts.
0