If we have a file with face centroid locations, how can we automatically get the nearest vertex?
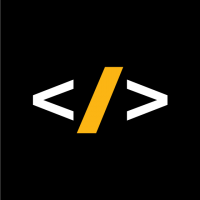
Erik Kostson
Member, Moderator, Employee Posts: 276
✭✭✭✭
Say we have a text file (called: vercentroids.txt) containing centroid locations (x,y,z coordinates) for different faces. We also have a face named selection that appears first on the Named selection tree. How can we automatically get the vertex nearest these centroid locations?
0
Best Answer
-
Below is a sample script showing one way of doing this. In summary it will read in the vercentroids text file, and loop through all faces in the first named selection and their vertices, trying to get the one closest to the centroid. Finally it will create a named selection containing the nearest vertices. As bonus it will create a stress result for each vertex.
import csv cx=[] cy=[] cz=[] myv=[] myvIds=[] dist=1E12 with open("D:/vercentroids.txt", 'rb') as f: # change file as needed reader = csv.reader(f) for row in reader: cx.append(row[0]) cy.append(row[1]) cz.append(row[2]) model = ExtAPI.DataModel.Project.Model analysis=model.Analyses[0] solution=analysis.Solution ns=model.NamedSelections.Children[0] for j in range(0,len(cx)): nId=ns.Location.Ids for face in nId: f = ExtAPI.DataModel.GeoData.GeoEntityById(face) vertices=f.Vertices for ver in vertices: vsx=ver.X vsy=ver.Y vsz=ver.Z dists=sqrt((float(cx[j])-vsx)**2+(float(cy[j])-vsy)**2+(float(cz[j])-vsz)**2) if dists<dist: myvs=(ver) dist=dists dist=1E12 myv.append(myvs) myvIds.append(myvs.Id) with Transaction(): for i in range(0,len(myvIds)): selection = ExtAPI.SelectionManager.CreateSelectionInfo(SelectionTypeEnum.GeometryEntities) selection.Ids=[myvIds[i]] newns=ExtAPI.DataModel.Project.Model.AddNamedSelection() newns.Location=selection r=solution.AddEquivalentStress() r.Location=newns
0
This discussion has been closed.