How to convert Ansys mechanical result file (.RST) to VTU/VTP format (typically used in paraview)
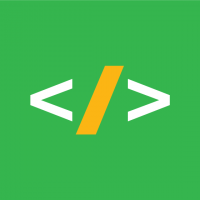
This code:
Extracts multiple fields (displacement and stress results) from an Ansys result file (.rst), and exports them to VTU format in a specified directory using DPF module
It then reads the VTU file using pyvista, extracts the surface mesh, and saves it as a VTP file.
These files can be used by SimAI
Comments
-
from ansys.dpf import core as dpf import pyvista as pv import os # Inputs rstpath = r"your path\file.rst" vtu_directory = r"D:/" vtu_file_name = "MyFile" # your file name # Initialize data source and model my_data_sources = dpf.DataSources(rstpath) my_model = dpf.Model(rstpath) # Get all available result names available_results = my_model.metadata.result_info.available_results result_names = [r.name for r in available_results if hasattr(dpf.operators.result, r.name)] #result_names = ["displacement_Y","stress_X","stress_Y"] #Either specify this or specify all results by commenting this line # Get mesh and time frequencies my_mesh = my_model.metadata.meshed_region my_time_scoping = ( dpf.operators.metadata.time_freq_provider(data_sources=my_data_sources) .outputs.time_freq_support.get_data() .time_frequencies.data_as_list ) # Define scoping (all nodes) my_scoping = dpf.Scoping() my_scoping.location = dpf.locations.nodal my_scoping.ids = my_mesh.nodes.scoping.ids # Extract result fields result_fields = [] for result_name in result_names: result_operator = dpf.operators.result.__getattribute__(result_name) result_field = result_operator( time_scoping=my_time_scoping, data_sources=my_data_sources, mesh=my_mesh, mesh_scoping=my_scoping ).outputs.fields_container.get_data()[0] result_fields.append(result_field) # Generate VTU file vtu_operator = dpf.operators.serialization.vtu_export( directory=vtu_directory, mesh=my_mesh, base_name=vtu_file_name ) for count, result_field in enumerate(result_fields): vtu_operator.connect(count + 3, result_field) vtu_operator.run() # Generate VTP file from VTU vtu_file_path = os.path.join(vtu_directory, vtu_file_name + "_T0000000-1.vtu") vtp_file_path = os.path.join(vtu_directory, vtu_file_name + "_surface.vtp") vtu = pv.read(vtu_file_path) vtp = vtu.extract_surface() vtp.save(vtp_file_path)
See below:
all results available in .RST is transferred in VTU and VTPIn case you have your own custom result file , you can create your own fields using them before writing a VTU/VTP file . Please refer below link to see how you can create your own custom field:
https://dpf.docs.pyansys.com/version/stable/examples/00-basic/03-create_entities.html#sphx-glr-examples-00-basic-03-create-entities-pyAlso if you want to just have solid elements and their results you can filter them out like below:
(This could be very useful for SimAI workflows, which allows only solid elements on VTU, ignoring CONTACT, SURF like elements and corresponding fields )# Filter to include only solid elements (using 'solid' as the shape type) solid_element_ids = [ elem.id for elem in my_mesh.elements#assuming you already have my_mesh from previously executed codes if elem.shape == "solid" ] # Define scoping for element ids of solid elements my_scoping = dpf.Scoping() my_scoping.location = dpf.locations.elemental my_scoping.ids = solid_element_ids #Creating mesh from solid element scopings my_mesh = dpf.operators.mesh.from_scoping(scoping=my_scoping,mesh=my_mesh).outputs.mesh.get_data() # Creating nodal scoping (all nodes of the solid elements only) my_scoping = dpf.Scoping() my_scoping.location = dpf.locations.nodal my_scoping.ids = my_mesh.nodes.scoping.ids
1 -
Hello Vishnu,
Thank you for this script, Can you provide same script for extracting modal analysis results to see all frequency?
0 -
@Vishnu said:
This code:Extracts multiple fields (displacement and stress results) from an Ansys result file (.rst), and exports them to VTU format in a specified directory using DPF module
It then reads the VTU file using pyvista, extracts the surface mesh, and saves it as a VTP file.
These files can be used by SimAI
Hello Vishnu,
Thank you for this script, Can you provide same script for extracting modal analysis results to see all frequency?
0 -
@Harikrushna are you interested in creating a VTU/VTP for your modal analysis?
what information would you need , just the modal frequencies? Typically the frequencies can be embedded by creating a new fieldlook at the below for how you can extract natural freq using DPF:
0 -
Yes, I want to create VTU/VTP file. I want to see them in paraview.
0