How do I automate a moving ImportedPressure object in Mechanical
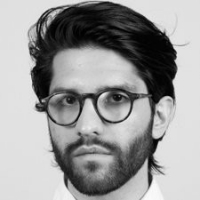
Aria
Member, Employee Posts: 67
✭✭✭✭
How do I generate an imported pressure load that moves as a function of time and vary the scale over time in Mechanical?
Tagged:
0
Best Answer
-
This is a sample code which generate n number of imported pressure loads, vary them over time and transform the load object as a function of time (points).
To run it, the Imported Load tree object needs to be selected.
import math ############ ## inputs ## time_range = [0,10] x_range = [5,50] # x0 to x1 y_range = [10,23] # y0 to y1 z_range = [-100,300] # z0 to z1 points = 10 ############ ############ def linspace(val_range, num): start, stop = val_range[0], val_range[1] diff = stop - start step = diff / (num - 1) return [start + step * i for i in range(num)] def sinus(x1, x2, num_points): return [math.sin(x1 + i * (x2 - x1) / num_points) for i in range(num_points)] imp = Tree.FirstActiveObject analysis = imp.Parent analysis.AnalysisSettings.NumberOfSteps = points xvals = linspace(x_range,points) yvals = linspace(y_range,points) zvals = linspace(z_range,points) scale_range = sinus(0,2*math.pi,points) for iter in range(0,points): with Transaction(suspendClicks=True): if iter == 0: load = imp.AddImportedPressure() else: load = load.Duplicate() table=load.GetTableByName("")[0] table[1] = iter+1 table[2] = round(scale_range[iter] ,2) load.PropertyByName("OriginX").InternalValue = float(xvals[iter]) load.PropertyByName("OriginY").InternalValue = float(yvals[iter]) load.PropertyByName("OriginZ").InternalValue = float(zvals[iter])
'''
0