How to replace a Group after an Imprint operation in SpaceClaim?
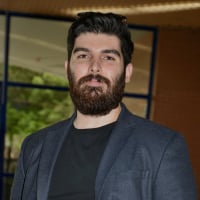
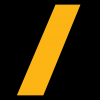
I have a Geometry in SCDM with a Solid body and a Surface which is overlapping with one of the Solid's faces. There's a Group / Named Selection that contains the Surface.
After an Imprint operation, I want to modify the Named Selection so that it contains the Solid's face created from the Imprint.
How can I do this in an Automated fashion?
Best Answer
-
You can use the script below to do this. The script identifies the solids and the surfaces, does the Imprint operation, and then compares the faces in each Named Selection with the faces of the Solid. If in the comparison the faces match - verified through coincident edges - a new Named Selection is created to replace the old one.
`
def get_solid_body():# Get the root part part = GetRootPart() # Get all the bodies in the entire part allBodies = part.GetAllBodies() #Find Solid body for body in allBodies: is_solid = body.GetMaster().Shape.IsClosed if is_solid: solidBody = body break return solidBody
def imprint_curves():
# Imprint Curves options = FixImprintOptions() options.Tolerance = MM(0.01) options.SearchFaces = True options.SearchEdges = True options.SearchCurves = True options.ImprintInstanceType = ImprintInstanceType.IgnoreInstances result = FixImprint.FindAndFix(options)
def replace_named_selections(targetBody):
# Get all Groups groups = WindowHelper.GetGroups() for group in groups: # Process each group / Named Selection groupName = group.GetName() print("Processing group: " + groupName) groupSelection = Selection.CreateByGroups(SelectionType.Primary, groupName) group_found = False for originFace in groupSelection.Items: # Iterate over face(s) included in the Group print("Origin Face: " + str(originFace)) found = False for targetFace in targetBody.Faces: print("Checking target face: " + str(targetFace)) if found: break # Check if all edges in originFace have a matching edge in targetFace noEdges = len(originFace.Edges) counter = 0 for originEdge in originFace.Edges: print("Origin Edge: " + str(originEdge)) for targetEdge in targetFace.Edges: print("Target Edge: " + str(targetEdge)) # Measure distances selection = EdgeSelection.Create([originEdge,targetEdge]) gapObj = MeasureHelper.MinDistanceBetweenObjects(selection) minDistanceObj = gapObj.Distance print("Min Distance (Objects):" + str(minDistanceObj)) gapAxes = MeasureHelper.MinDistanceBetweenAxes(selection) minDistanceAxes = gapAxes.Distance print("Min Distance (Axes):" + str(minDistanceAxes)) # Check if edges match if minDistanceObj < 1e-16 and minDistanceAxes < 1e-16: counter += 1 print('One match found') break if counter == noEdges: found = True break if found and counter == noEdges: # Delete the original named selection group result = NamedSelection.Delete(groupName) # Create new Named Selection with targetFace primarySelection = FaceSelection.Create(targetFace) secondarySelection = Selection.Empty() result = NamedSelection.Create(primarySelection, secondarySelection) # Get the newly created group and rename it newGroup = WindowHelper.GetGroups()[-1] result = NamedSelection.Rename(newGroup.GetName(), groupName) print("Named selection replaced") group_found = True break # Break out of the inner loop once the named selection is replaced if group_found: break # Move on to the next group if the named selection was replaced
tBody = get_solid_body()
result = imprint_curves()
result = replace_named_selections(tBody)
`0