How do I get the location of the mouse cursor in the graphics window?
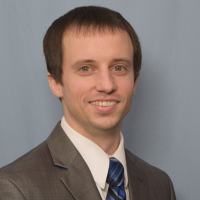
I'm trying to allow the user to draw a rectangle on the screen that remains stationary as the model is moved, rotated, etc. Looking here (link), this code will draw a line from (0, 0) to (100, 100):
Point2D = Graphics.CreatePixelPoint
l1 = Graphics.Scene.Factory2D.CreatePolyline([Point2D(200, 200), Point2D(200, 300), Point2D(300, 300), Point2D(300, 200)])
l1.Closed = True
I'm now trying to get the location of a user's mouse click in the graphics window to enable him or her to specify the corners of the box. If I knew the dimensions and location on the screen of the graphics window, I think I could get the location of the mouse on the screen and work out from there the locations of the corners of the box. However, I don't know how to get the dimensions and location on the screen of the graphics window. Further, I think there's a decent chance that I'm missing something, and there's a means of directly obtaining the location of the cursor in the graphics window (i.e., without doing the math to convert the screen location of the mouse to the graphics window location).
Best Answers
-
@tlewis3348 I am not sure if there is an exposed API for that. A workaround I can think of is that you create a Windows Forms that overlays the Graphics Scene and would close on the 4th mouse click. You can control the transparency of the Form too.
import clr clr.AddReference('System.Windows.Forms') from System.Windows.Forms import Application, Form, FormStartPosition from System.Drawing import Point, Size Pane = ExtAPI.UserInterface.GetPane(MechanicalPanelEnum.Graphics) width = Pane.ControlUnknown.width height = Pane.ControlUnknown.height left = Pane.CommandContainer.WindowRect.Left top = Pane.CommandContainer.WindowRect.Top command_bar_h = Pane.CommandContainer.WindowRect.Height class TransparentForm(Form): def __init__(self): self.click_count = 0 self.Text = "Transparent Form" self.FormBorderStyle = 0 self.BackColor = System.Drawing.Color.Lime self.Opacity = 0.05 self.MouseClick += self.on_mouse_click self.StartPosition = FormStartPosition.Manual self.Location = Point(left, top) self.Size = Size(width, height + command_bar_h) def on_mouse_click(self, sender, event): x, y = event.X, event.Y print("Mouse position: (%s, %s)" % (x, y)) self.click_count += 1 if self.click_count >= 4: self.Close() form = TransparentForm() Application.Run(form)
1 -
@Ayush Kumar Yes, I'm aware of that possibility, but I would still need to know the size and location of the graphics window in order to know the location of the box relative to the model. Is there a means of getting that?
0 -
@tlewis3348, updated the code above with Graphic windows position and dimensions, hope this helps!
1
Answers
-
@AKD-Scripting-Team does someone know whether this is feasible ?
1 -
@Pernelle Marone-Hitz I'm just checking to see if the @AKD-Scripting-Team has said anything about whether this is feasible.
0 -
@Ayush Kumar Thanks! That's very helpful.
1