Wrong calculation result when using imported user module?
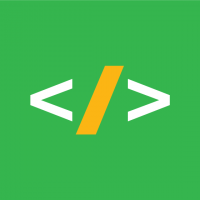
Hello,
I am trying to develop a script to do some fatigue calculations, but I noticed something weird and wanted to investigate further.
Hi,
I noticed when I use a function from an imported user module, the result is for some reason different than if I run the same function from the module itself.
I have following code which is a part of a module I will import into the main script:
```
from module_base import * #This will import the Ansys.Mechanical.DataModel.Enums items
import math #another modules you are using in the project
def Initialize(MyExtAPI, MyAnsys):
global ExtAPI; global Ansys
ExtAPI = MyExtAPI; Ansys = MyAnsys
def CalculateFatigueLife_DNV_Test(stress_range, SN_Curve,thickness,SCF=1,ref_thickness=25):
m1=3
log_a1=12.192
m2=5
log_a2=16.32
k=0.05
trans=1000000
log_N=log_a1-m1*math.log10(stress_range*SCF*math.pow(thickness/ref_thickness,k)) N = math.pow(10,log_N) if(N>trans): log_N=log_a2-m2*math.log10(stress_range*SCF*math.pow(thickness/ref_thickness,k)) N = math.pow(10,log_N) return N
print(CalculateFatigueLife_DNV_Test(334.7,"C CP",30,1,25))
import math
import Ansys.ACT.Math as math_ACT
import mech_dpf
import Ans.DataProcessing as dpf
import sys
CodePath = r"D:\ANSYS Scripts"
if not CodePath in sys.path:
sys.path.append(CodePath)
import FatigueFunctions as FF #module with fatigue calculations functions
reload(FF)
FF.Initialize(ExtAPI, Ansys)
print(FF.CalculateFatigueLife_DNV(334.7,"C CP",30,1,25))
print("Test: "+repr(FF.CalculateFatigueLife_DNV_Test(334.7,"C CP",30,1,25)))
FF.Initialize(ExtAPI, Ansys)
print("Test: "+repr(FF.CalculateFatigueLife_DNV_Test(334.7,"C CP",30,1,25)))
```
The code above prints: "Test: 41498.544"
I don't understand why when I call the function from the main code I get the different result from running the same function from the imported module. Is this a bug? Did anyone come across this before? My end plan here is to develop a module I can import into other scripts to carry out calculations etc.
Thanks
Running the main script yields the following message
Comments
-
Something went wrong with the formatting in my post, I apologise, please see the below for clarity.
Imported module:
from module_base import * #This will import the Ansys.Mechanical.DataModel.Enums items import math #another modules you are using in the project def Initialize(MyExtAPI, MyAnsys): global ExtAPI; global Ansys ExtAPI = MyExtAPI; Ansys = MyAnsys def CalculateFatigueLife_DNV_Test(stress_range, SN_Curve,thickness,SCF=1,ref_thickness=25): m1=3 log_a1=12.192 m2=5 log_a2=16.32 k=0.05 trans=1000000 print(CalculateFatigueLife_DNV_Test(334.7,"C CP",30,1,25))
The above produces message: "40379" (and some extra digits
Main code:
import math import Ansys.ACT.Math as math_ACT import mech_dpf import Ans.DataProcessing as dpf import sys CodePath = r"D:\ANSYS Scripts" if not CodePath in sys.path: sys.path.append(CodePath) import FatigueFunctions as FF #module with fatigue calculations functions reload(FF) FF.Initialize(ExtAPI, Ansys) print("Test: "+repr(FF.CalculateFatigueLife_DNV_Test(334.7,"C CP",30,1,25)))
The above produces following message: "Test: 41498" (and again, some extra digits)
0 -
The issue is that the thickness and ref_thickness arguments are both being defined as integers, which causes
thickness/ref_thickness
to evaluate as 1 (an integer) instead of 1.2. I am not sure why we don't see this behavior when running the function directly in Mechanical, but luckily this allowed you to catch the bug.A quick fix is to add this line to your function:
def CalculateFatigueLife_DNV_Test(stress_range, SN_Curve,thickness,SCF=1,ref_thickness=25): thickness = float(thickness) ...
1 -
I did a little more digging. The Mechanical scripting environment seems to always return a float from integer division. This is not the default behavior for IronPython 2.7 (which is what Mechanical runs). I suspect that the Mechanical initialization scripts include something like:
from __future__ import division
An alternative solution is to add the above line to the top of your FatigueFunctions.py file.
0 -
I have had issues myself with using integers to do math operations and not remembering that they are different from a float. It would be best practice to code your math functions with a float(SomeVal) function to ensure the input values are actually float numbers and not integers when appropriate.
1 -
@Landon Mitchell Kanner , casting thickness as float seems to have solved this issue, The results are now matching the calculations. I will add some comments into the code to remember about this behaviour in the future. Thanks for help.
1