How can I create copies of the meshed region to plot multiple field contours on the duplicated mesh
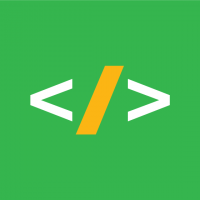
Vishnu
Member, Employee Posts: 222
✭✭✭✭
Sometimes, we simply want to create a linear pattern of the existing mesh region in DPF to plot various contours on them.
Tagged:
0
Comments
-
Below is the python result implementation of contour plot of displacement in X,Y and Z on the duplicated meshed regions. Note for larger mesh size this could be cumbersome to do it.
def post_started(sender, analysis):# Do not edit this line define_dpf_workflow(analysis) # Uncomment this function to enable retrieving results from the table/chart # def table_retrieve_result(value):# Do not edit this line # import mech_dpf # import Ans.DataProcessing as dpf # wf = dpf.Workflow(this.WorkflowId) # wf.Connect('contour_selector', value) # this.Evaluate() def define_dpf_workflow(analysis): import mech_dpf import Ans.DataProcessing as dpf #Result Data analysis1 = ExtAPI.DataModel.Project.Model.Analyses[0] dataSource = dpf.DataSources(analysis1.Solution.ResultFilePath) #model my_model = dpf.Model(analysis1.Solution.ResultFilePath) #my mesh my_mesh = my_model.Mesh #extract field dispfield1 = dpf.operators.result.displacement_X(data_sources=dataSource).outputs.fields_container.GetData()[0] dispfield2 = dpf.operators.result.displacement_Y(data_sources=dataSource).outputs.fields_container.GetData()[0] dispfield3 = dpf.operators.result.displacement_Z(data_sources=dataSource).outputs.fields_container.GetData()[0] #Inputs total_copies = 2 list_of_fields = [dispfield1,dispfield2,dispfield3] offsets_for_copies = [20.0,0.0,0.0] def create_copy_mesh_field(list_of_fields,total_copies): #check if size matches if total_copies + 1 > list_of_fields.Count: list_of_fields.extend([list_of_fields[0]] * (total_copies + 1 - len(list_of_fields))) mesh_nodes = my_mesh.Nodes mesh_node_ids = my_mesh.NodeIds mesh_elements = my_mesh.Elements total_nodes = mesh_nodes.Count total_elements = mesh_elements.Count for copy_nr in range(1,total_copies+1): offsetX = offsets_for_copies[0]*copy_nr offsetY = offsets_for_copies[1]*copy_nr offsetZ = offsets_for_copies[2]*copy_nr list(map(lambda x: my_mesh.AddNode(total_nodes * copy_nr + x[0] + 1, [x[1].X + offsetX, x[1].Y + offsetY, x[1].Z + offsetZ]), enumerate(mesh_nodes))) updated_mesh_nodes = my_mesh.NodeIds # Create a dictionary to map node IDs to their indices index_mapping = {value: index for index, value in enumerate(updated_mesh_nodes)} # Use map and lambda to add elements list(map(lambda x: my_mesh.AddSolidElement( total_elements * copy_nr + x[0] + 1, [index_mapping[node_id + total_nodes * copy_nr] for node_id in x[1].NodeIds if node_id + total_nodes * copy_nr in index_mapping] ), enumerate(mesh_elements))) com_field_size = my_mesh.NodeCount combined_field = dpf.FieldsFactory.CreateScalarField(com_field_size) combined_fieldData = [] for field in list_of_fields: combined_fieldData = combined_fieldData + [field.GetEntityDataById(x)[0] for x in mesh_node_ids] combined_field.Data = combined_fieldData combined_field.ScopingIds = my_mesh.NodeIds return my_mesh,combined_field my_mesh,combined_field = create_copy_mesh_field(list_of_fields,total_copies) dpf_workflow = dpf.Workflow() # dpf_workflow.SetInputName(u, 0, 'time') # dpf_workflow.Connect('time', timeScop) dpf_workflow.SetOutputMesh(my_mesh) dpf_workflow.SetOutputContour(combined_field) dpf_workflow.Record('wf_id', False) this.WorkflowId = dpf_workflow.GetRecordedId()
2