How to extract contact gap using PyDPF
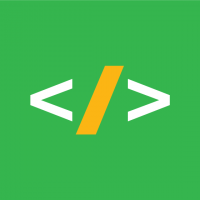
A similar procedure could be adapted for mechdpf using python results as well.
The method below uses getattribute which makes the code very generic for extraction of various result fields.
We can typically modify the result_name (in line 8) while keeping the rest of the code intact, effectively serving as a template.
Comments
-
import matplotlib.pyplot as pyplot from ansys.dpf import core as dpf from ansys.dpf.core.plotter import DpfPlotter # Inputs rstpath = r"Your path to \file.rst" result_name = "contact_gap_distance" # data source my_data_sources = dpf.DataSources(rstpath) # model my_model = dpf.Model(rstpath) # mesh my_mesh = my_model.metadata.meshed_region # Time List my_time_scoping = ( dpf.operators.metadata.time_freq_provider(data_sources=my_data_sources) .outputs.time_freq_support.get_data() .time_frequencies.data_as_list ) # all available results all_results = dir(dpf.operators.result) # Get result operator result_operator = dpf.operators.result.__getattribute__(result_name) # Field Containers at ns result_field_container = result_operator( time_scoping=1, data_sources=my_data_sources, mesh=my_mesh, ).outputs.fields_container.get_data() # Field Containers at all mesh result_field_container = result_operator( time_scoping=my_time_scoping, data_sources=my_data_sources, mesh=my_mesh ).outputs.fields_container.get_data() #convrting to nodalresults result_field_container = dpf.operators.averaging.to_nodal_fc( fields_container=result_field_container, mesh=my_mesh, ).outputs.fields_container.get_data() # First Field usually field corresponding to first time scoping resultfield = result_field_container[0] # Chain Multiple operators here if you want########example below # minmax min_max_op = dpf.operators.min_max.min_max_fc(result_field_container) min_field = min_max_op.outputs.field_min() max_field = min_max_op.outputs.field_max() # Scoping nodes or elements result_scoping = resultfield.scoping.ids # print result at all scopings and results corresponding to it result_scoping.sort() for scoping in result_scoping: try: print( "result at scoping -->", scoping, "is -->", resultfield.get_entity_data_by_id(scoping), ) except: print("issue in extracting result for -->", scoping) # plot the field on your mesh pl = DpfPlotter() pl.add_mesh(my_mesh) pl.add_field(resultfield, show_max=True, show_min=True) pl.show_figure() # graph plot using matplotlib pyplot.plot(my_time_scoping, max_field.data_as_list, "ro", label="Max") pyplot.plot(my_time_scoping, min_field.data_as_list, "bo", label="Min") pyplot.xlabel("Time (s)") pyplot.ylabel("Result (unit_of_result)") pyplot.legend() pyplot.show()
0 -
@Vishnu ,
Seems like there is an operator for this: op = dpf.operators.result.contact_gap_distance()Why are you using the more obscure method of:
result_operator = dpf.operators.result.getattribute(result_name)Is there a difference in what you are doing vs. the documented operator?
0 -
@Mike.Thompson
This method tends to be quite generic. The customer can usually modify the result_name (in line 8) while keeping the rest of the code intact, effectively serving as a template for users.0