How to speed up command snippet creation and create a progress bar ?
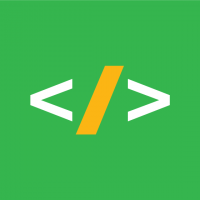
Hi,
Thanks to this post :
https://developer.ansys.com/blog/script-tip-friday-efficiently-combining-mapdl-and-mechanical-scripting
I wrote a similar function, with minor adjustments.
My problem is : When the model contains lots of joints, the creation time is long ... very long.
My questions are :
1- Is there a way to speed up this function ? (I try to add "with Transaction(suspendClicks=True):", it seems to be better, but not very fast)
2- Is there a way to create a progress bar in order to see the progression ?
def def_creation_ID(analysis): with Transaction(suspendClicks=True): connections_folder = ExtAPI.DataModel.Project.Model.Connections joints_temp = connections_folder.GetChildren(DataModelObjectCategory.Joint,True) joints = [joint for joint in joints_temp if joint.Suppressed == False and len(joint.MobileLocation.Ids) != 0 and len(joint.ReferenceLocation.Ids) != 0] nb_joints = 0 for j in joints: if j.Suppressed is False: nb_joints += 1 with Transaction(suspendClicks=True): nb_insert_command = len(j.Children) if nb_insert_command > 0: for i in range(nb_insert_command): if j.Children[i].Name == r"commande_joint_ID": j.Children[i].Delete() new_snippet = j.AddCommandSnippet() new_snippet.Name = r"commande_joint_ID" string1 = 'joint_'+str(nb_joints) + ' = _jid \n' string2 = '*DIM,joint_name_'+str(nb_joints)+',string,248 \n' string3 = 'joint_name_'+str(nb_joints) + '(1) = \'' + j.Name + '\'\n' string4 = '*DIM,joint_type_'+str(nb_joints)+',string,248 \n' string5 = 'joint_type_'+str(nb_joints) + '(1) = \'' + str(j.Type) + '\'\n' new_snippet.AppendText(string1) new_snippet.AppendText(string2) new_snippet.AppendText(string3) new_snippet.AppendText(string4) new_snippet.AppendText(string5) new_snippet.AppendText("nb_joints = " + str(nb_joints)) ExtAPI.DataModel.Tree.Refresh()
Answers
-
For speeding up:
having Transaction within transaction won't help. I have made a few changes based on best of my knowledge. Please adopt it for your case.
- Avoid Adding/Removing of objects in tree a lot.
- Minimize AppendText, Concatenate strings and AppendText once.
import time start_time = time.time() with Transaction(suspendClicks=True): connections_folder = ExtAPI.DataModel.Project.Model.Connections joints_temp = DataModel.GetObjectsByType(DataModelObjectCategory.Joint) joints = [joint for joint in joints_temp if joint.Suppressed == False] nb_joints = 0 new_snippet = None for j in joints: if j.Suppressed is False: nb_joints += 1 insert_new = True string1 = 'joint_'+str(nb_joints) + ' = _jid \n' string2 = '*DIM,joint_name_'+str(nb_joints)+',string,248 \n' string3 = 'joint_name_'+str(nb_joints) + '(1) = \'' + j.Name + '\'\n' string4 = '*DIM,joint_type_'+str(nb_joints)+',string,248 \n' string5 = 'joint_type_'+str(nb_joints) + '(1) = \'' + str(j.Type) + '\'\n' snippet_to_define = "".join([string1, string2,string3, string4, string5]) new_snippet = None nb_insert_command = len(j.Children) if nb_insert_command > 0: for chld in j.Children: if chld.Name == "commande_joint_ID": chld.Input = "" new_snippet = chld insert_new = False if insert_new: new_snippet = j.AddCommandSnippet() new_snippet.Name = r"commande_joint_ID" new_snippet.AppendText(snippet_to_define) if new_snippet: new_snippet.AppendText("nb_joints = " + str(nb_joints)) ExtAPI.DataModel.Tree.Refresh() end_time = time.time() print(end_time - start_time)
0 -
For Progress Object:
Here is an example:
ID_CDBCONVERT: Is dummy input.
Please it uses internalObject which may change in future and it is not supported.
def step_function(): pass progressObj = Ansys.Mechanical.Application.Progress() dlg = ExtAPI.Application.ScriptByName("jscript").CallJScript("doStartProgress", ["ID_CDBCONVERT", progressObj.InternalObject]) Wait(100) progressObj.SetProgress(5,'Test',50,'Processing Current Step') ExtAPI.Application.InvokeBackground(step_function, progressObj)
0 -
@Jean
You can use this to delete multiple objects with one line. It is more efficient then the individual Object.Delete() methods. You can loop through objects and instead of deleting them, simply add them to a list of objects to delete later with this single call.Also, you may want to think about what you need this all for. It seems you are trying to track/match the mechanical joints to the APDL joint types. You can use the Solution.SolverData object to get the APDL element types of any given joint after the solve process. If this is being used for some post processing, this may be a cleaner way to dynamically get the info you need in the background of the post code. Perhaps you can avoid the code snippet creations all together and make the processing time moot.
ObjsToDelete -> Object list you want to delete
ExtAPI.DataModel.Remove(ObjsToDelete)
1 -
Thanks for all your answers, I will try them.
@Mike.Thompson said:
@Jean
You can use this to delete multiple objects with one line. It is more efficient then the individual Object.Delete() methods. You can loop through objects and instead of deleting them, simply add them to a list of objects to delete later with this single call.Also, you may want to think about what you need this all for. It seems you are trying to track/match the mechanical joints to the APDL joint types. You can use the Solution.SolverData object to get the APDL element types of any given joint after the solve process. If this is being used for some post processing, this may be a cleaner way to dynamically get the info you need in the background of the post code. Perhaps you can avoid the code snippet creations all together and make the processing time moot.
ObjsToDelete -> Object list you want to delete
ExtAPI.DataModel.Remove(ObjsToDelete)
Hi Mike,
Thanks for your suggestion.
I tried to use "Solution.SolverData" and it works very well. But I have a problem with this method, we can't use it in a spectrum analysis (or maybe you have a solution and it will be a wonderful).
That why I use this command snippet...0 -
@Jean ,
Is the functional goal to do post processing of joints for a response spectrum analysis? Get the XYZ forces, and cross reference the element ids for APDL to Mechanical?0 -
@Mike.Thompson
Yes, I would like to get reaction forces and moments of all joints in order to post processing them.
We often do this process :
1- get joint reactions on static analysis
2- get joint reactions on spectrum analysis
3- calculate (1+2) and (1-2)
4- with Excel files we have, verifying if the joint (bolt for example) is ok or not.For step 1, the "Solution.SolverData" could worked.
For step 2, I didn't fing the way to get reactions.
For step 3 and 4, we would like to get the type of the joint and the name, in order to do some checks with the model.0 -
Are you getting forces and moments with FSUM APDL command in RS analysis?
0 -
This is an example of how to get a .csv file of beams in a random vib analysis. With a slight modification you can convert it to joints and RS analysis. There is an APDL command snippet that will use FSUM and write a text file. This is then parsed with a python code object under the solution and a new .csv file is written with mechanical info. Easier and less objects than a snippet under each joint.
0 -
I am getting forces and moments with FSUM APDL command in RS analysis.
It works, but the workflow is not user friendly.
Thanks a lot for the model you shared. I will look at it and see if I can adapt it for joints.0