How to plot a custom chart using ACT Console?
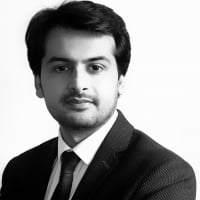
Ayush Kumar
Member, Moderator, Employee Posts: 467
✭✭✭✭
Answers
-
This can be done using
System.Windows.Forms.DataVisualization.Charting
Example project + Code: CustomChart.zip
Example code: This code will require modification as per user requirement
# Import the library import clr clr.AddReference("System.Drawing") clr.AddReference("System.Windows.Forms") clr.AddReference("System.Windows.Forms.DataVisualization") from System.Drawing import * from System.Windows.Forms import * class PlotHarmonicResponse(Form): def __init__(self): self.plot() def plot(self): # Set chart properties chart = DataVisualization.Charting.Chart() chart_area = DataVisualization.Charting.ChartArea() chart_area.AxisX.Title = "Frequency [Hz]" chart_area.AxisY.Title = "Deflection [m]" # Define a series for each curve to be added to the chart series_1 = DataVisualization.Charting.Series() series_1.Color = Color.Green series_1.Name = "Limit Curve" series_1.ChartType = DataVisualization.Charting.SeriesChartType.Line frequencies=[40, 50, 1000, 20000, 40000] for index, freqs in enumerate(frequencies): series_1.Points.AddXY(index, freqs) # Add series to the chart chart.Series.Add(series_1) # Add chart areas to chart chart.ChartAreas.Add(chart_area) # Add chart to the application self.Controls.Add(chart) # Create plot Application.EnableVisualStyles() plot_response = PlotHarmonicResponse() Application.Run(plot_response)
Detailed documentation: https://docs.microsoft.com/en-us/dotnet/api/system.windows.forms.datavisualization.charting?view=netframework-4.8
0