How to plot shell data at different integration points for LSDYNA using PyDPF?
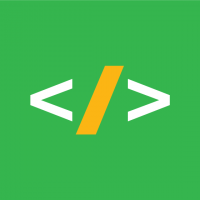
DavidTR
Member, Employee Posts: 4
✭✭✭
in Structures
How to plot shell data at different integration points for LSDYNA using PyDPF?
0
Comments
-
Here is an example using PyDPF which also includes other types of results like animations and plots.
Paths must be updated accordingly.
- import matplotlib.pyplot as plt
- from ansys.dpf import core as dpf
- from ansys.dpf.core.plotter import DpfPlotter
- """Adding an r or double \\ should fix path issues"""
- d3plot_path = r"D:\PATHTOD3PLOT\d3plot"
- """ACTunits file gets generated when using WB-LSDYNA to solve the analysis. It can be created manually too"""
- units_path = r"D:\PATHTOACTUNITS\name.actunits"
- ds = dpf.DataSources()
- ds.set_result_file_path(d3plot_path, "d3plot")
- ds.add_file_path(units_path, "actunits")
- model = dpf.Model(ds)
- print(model)
- """ model.plot() # Plots the mesh"""
- """5 is the d3plot state"""
- stress_vm = model.results.stress_von_mises(time_scoping=[5])
- disp = model.results.displacement(time_scoping=[5])
- fields = disp.outputs.fields_container()
- """2 is the 3rd displacement component, i.e. Z"""
- field = fields.select_component(2)[0]
- mesh = model.metadata.meshed_region
- meshes = dpf.operators.mesh.split_mesh(mesh=mesh, property="eltype").eval()
- print(meshes)
- print(meshes[0])
- print(meshes[1])
- disp_f = model.results.displacement(time_scoping=[5]).eval()
- scop = dpf.Scoping(ids=[1], location=dpf.locations.nodal)
- stress_op = model.results.stress(time_scoping=[5])
- disp_f_solid = model.results.displacement(time_scoping=[5], mesh_scoping=meshes[1].nodes.scoping).eval()
- print(disp_f_solid[0])
- disp_f_shell = model.results.displacement(time_scoping=[5], mesh_scoping=meshes[0].nodes.scoping).eval()
- print(disp_f_shell[0])
- stress_f_solid = model.results.stress(time_scoping=[5], mesh_scoping=meshes[1].elements.scoping).eval()
- stress_f_solid_x = stress_f_solid.select_component(0)
- print(stress_f_solid[0])
- stress_f_shell = model.results.stress(time_scoping=[5], mesh_scoping=meshes[0].elements.scoping).eval()
- """It seems to be wrong in the documentation - Top=3 Bot=0 Mid=1"""
- stress_f_shell_top = dpf.operators.utility.change_shell_layers(fields_container=stress_f_shell, e_shell_layer=3,
- mesh=meshes[0]).eval()
- stress_f_shell_top_x = stress_f_shell_top.select_component(0)
- print(stress_f_shell[0])
- pl = DpfPlotter()
- pl.add_field(stress_f_solid_x[0], meshes[1], deform_by=disp_f_solid[0])
- pl.add_field(stress_f_shell_top_x[0], meshes[0], deform_by=disp_f_shell[0])
- pl.show_figure()
- sargs_shell = dict(title="Stress X Shell Top", fmt="%.3e", title_font_size=20, label_font_size=16)
- stress_f_shell_top[0].plot(deform_by=disp_f_shell[0], scalar_bar_args=sargs_shell)
- sargs = dict(title="Displacement", fmt="%.3e", title_font_size=20, label_font_size=16)
- u_all = model.results.displacement.on_all_time_freqs.eval()
- u_all.animate(deform_by=u_all, save_as=r"D:\PATHTOSAVEANIMATION\name.gif",
- scalar_bar_args=sargs)
- """These come from the d3plot file"""
- K = model.results.global_kinetic_energy().eval()
- U = model.results.global_internal_energy().eval()
- H = model.results.global_total_energy().eval()
- plt.plot(K.time_freq_support.time_frequencies.data, K[0].data, label="Kinetic")
- plt.plot(U.time_freq_support.time_frequencies.data, U[0].data, label="Internal")
- plt.plot(H.time_freq_support.time_frequencies.data, H[0].data, label="Total")
- plt.xlabel("Time ({:s})".format(K.time_freq_support.time_frequencies.unit))
- plt.ylabel("Energies ({:s})".format(K[0].unit))
- plt.legend()
- plt.show()
- binout_path = r"D:\PATHTOBINOUT\binout0000"
- dp = dpf.DataSources()
- dp.set_result_file_path(binout_path, "binout")
- dp.add_file_path(units_path, "actunits")
- model_binout = dpf.Model(dp)
- """These come from the binout file"""
- part_sco = dpf.Scoping(ids=[1, 2], location="part")
- PKE_op = model_binout.results.part_kinetic_energy()
- PKE_op.inputs.entity_scoping.connect(part_sco)
- PKE = PKE_op.eval()
- PIE_op = model_binout.results.part_internal_energy()
- PIE_op.inputs.entity_scoping.connect(part_sco)
- PIE = PIE_op.eval()
- rescope_op = dpf.operators.scoping.rescope()
- rescope_op.inputs.fields.connect(PKE.time_freq_support.time_frequencies)
- rescope_op.inputs.mesh_scoping.connect(PKE[0].scoping)
- t_field = rescope_op.outputs.fields_as_field()
- t_vals = t_field.data
- plt.plot(t_vals, PKE.get_field({"part": 1}).data, label="Kinetic, Part 1")
- plt.plot(t_vals, PKE.get_field({"part": 2}).data, label="Kinetic, Part 2")
- plt.plot(t_vals, PIE.get_field({"part": 1}).data, label="Internal, Part 1")
- plt.plot(t_vals, PIE.get_field({"part": 2}).data, label="Internal, Part 2")
- plt.xlabel("Time ({:s})".format(t_field.unit))
- plt.ylabel("Energy ({:s})".format(PIE.get_field({"part": 1}).unit))
- plt.legend()
- plt.show()
1