How to edit any legend value with a UI box in Mechanical?
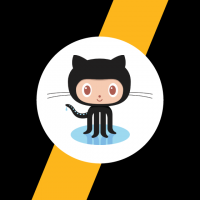
There are some issues pertaining to editing contour legend values in Mechanical. One solution is to add the user environmental variable in Windows, ANS_USE_OPENGL_LEGEND_EDIT to 1, from 2024R2. But, if it doesn't work, how can I create a python button in Mechanical that allows me to edit any contour legend value?
Best Answer
-
This is a continuation of a previous post by @Aria where UI was created via Python button for Contour Max and Min. Thanks Aria for this example!
The current solution expands further generalizing for any contour legend value, where the UI dynamically changes based on number of contour bands.
The below code can be run from Mechanical scripting console, or can be added into a Python button inside Mechanical using Button Editor (In Mechanical --> Automation Tab --> Manage --> Paste the code and save it after giving the button a name).
Once the button is created, here are the steps to use.
1. Activate any particular result object where contour is to be updated (say Equivalent Stress as shown below).- Click on the button we created earlier. Pop up box appears. Let's say I want to change the 3rd value from the top (labeled as Legend Value 3 in the pop up box) from default value to say 21
- Change the value to 21 and click on "Update Contour" at the bottom of the pop up box. Value will be updated.
import clr clr.AddReference("Ans.Utilities") clr.AddReference('Ans.UI.Toolkit') clr.AddReference('Ans.UI.Toolkit.Base') import Ansys.UI.Toolkit import Ansys.UI.Toolkit.Base def ChangeContours(): try: example = ContourGUI() example.Run() except: ExtAPI.Log.WriteError("Exception in ChangeContours()") def GetMinMax(): try: legendSettings = Ansys.Mechanical.Graphics.Tools.CurrentLegendSettings() noBands = legendSettings.NumberOfBands legendData = [] for i in range(noBands): legendData.append(legendSettings.GetUpperBound(noBands - i - 1).Value) legendData.append(legendSettings.GetLowerBound(0).Value) print(legendData) return legendData, noBands except: ExtAPI.Log.WriteError("Exception in GetMinMax()") class WindowUI(Ansys.UI.Toolkit.Window): aLabel = None aButton = None aTextBox = None mainPanel = None text_boxes = [] # List to store text boxes def __init__(self): self.Location = Ansys.UI.Toolkit.Drawing.Point(100,100) self.Text = 'Contour editor' self.Size = Ansys.UI.Toolkit.Drawing.Size(250,150) self.StatusBar.Visible = False self.__BuildUI() #this will be defined later self.BeforeClose += Ansys.UI.Toolkit.WindowCloseEventDelegate(self.Window_Close) def __BuildUI(self): res_unit = Tree.FirstActiveObject.Maximum.Unit self.mainPanel = Ansys.UI.Toolkit.TableLayoutPanel() self.Add(self.mainPanel) self.mainPanel.Columns.Add(Ansys.UI.Toolkit.TableLayoutSizeType.Percent, 100) self.mainPanel.Rows.Add(Ansys.UI.Toolkit.TableLayoutSizeType.Percent, 33) self.mainPanel.Rows.Add(Ansys.UI.Toolkit.TableLayoutSizeType.Percent, 34) self.mainPanel.Rows.Add(Ansys.UI.Toolkit.TableLayoutSizeType.Percent, 33) legendData, noBands = GetMinMax() for i in range(noBands+1): label = 'Legend Val ' + str(i+1) + '[' + res_unit + ']' self.aLabel = Ansys.UI.Toolkit.Label(label) self.mainPanel.Controls.Add(self.aLabel, i, 0) self.aTextBox = Ansys.UI.Toolkit.TextBox() self.aTextBox.Text = legendData[i].ToString() self.mainPanel.Controls.Add(self.aTextBox, i, 1) self.text_boxes.append(self.aTextBox) self.aButton = Ansys.UI.Toolkit.Button('Update Contour') self.aButton.Click += Ansys.UI.Toolkit.EventDelegate(self.On_Click) self.mainPanel.Controls.Add(self.aButton, i+1, 0) def On_Click(self, sender, args): global textString # Here I update the legend res_unit = Tree.FirstActiveObject.Maximum.Unit legendSettings = Ansys.Mechanical.Graphics.Tools.CurrentLegendSettings() noBands = legendSettings.NumberOfBands # Loop over the text_boxes to get each input value for i, textBox in enumerate(self.text_boxes): textString = textBox.Text if ',' in textString: textString = textString.Replace(',','.') value = float(textString) try: legendSettings.SetUpperBound(noBands - i - 1,Quantity(value, res_unit)) except: legendSettings.SetLowerBound(0,Quantity(value, res_unit)) def Window_Close(self, sender, args): Ansys.UI.Toolkit.Application.Quit() class ContourGUI: ex1 = None def __init__(self): self.ex1 = WindowUI() def Run(self): Ansys.UI.Toolkit.Application.Initialize() self.ex1.Show() Ansys.UI.Toolkit.Application.Run() ChangeContours()
2