How to create a custom Frequency Response chart?
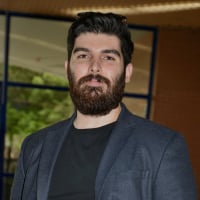
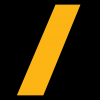
I'm working with a Harmonic Analysis system and have two deformation Frequency Response charts that I want to divide with one another to create a custom one. How can I do this?
Best Answer
-
Creating a custom Frequency Response chart is not available in Mechanical. However, you can approach this by using either a Line Chart object or through a matplotlib plot.
Line Chart
The idea is to extract the data from the two result objects, create the custom dataset, write the new data in a csv, and use that csv to plot in Mechanical through a Line Chart object. The script which can be executed with IronPython:import csv import Ansys.Mechanical.DataModel.Utilities as a ### Get Frequency Reponse object data ### respX = DataModel.GetObjectsByName('Frequency Response - X Deform')[0] respZ = DataModel.GetObjectsByName('Frequency Response - Z Deform')[0] tabDataX = respX.TabularData freqDataX = list(tabDataX['Frequency']) ampDataX = list(tabDataX['Amplitude']) phaseDataX = list(tabDataX['Phase Angle']) tabDataZ = respZ.TabularData freqDataZ = list(tabDataZ['Frequency']) ampDataZ = list(tabDataZ['Amplitude']) phaseDataZ = list(tabDataZ['Phase Angle']) freqData = freqDataX #Same frequency range for both results ampData = [aX / aZ for aX, aZ in zip(ampDataX, ampDataZ)] ### Create csv source file ### csvAmp = r"D:\outputAmp.csv" dataAmp = zip(freqData, ampData) with open(csvAmp, 'wb') as file: writer = csv.writer(file, delimiter='\t') writer.writerow(['Frequency ', 'Amplitude ']) writer.writerow(['Hz', 'mm']) writer.writerows(dataAmp) ### Select / Create Line Chart and use new source ### solObj = DataModel.Project.Model.Analyses[0].Solution resNames = [res.Name for res in solObj.Children] ampName = 'Line Chart - Custom Amplitude' # Select / Create Line Chart if ampName not in resNames: lineAmp = solObj.AddLineChart2D() lineAmp.Name = ampName else: lineAmp = solObj.Children[resNames.index(ampName)] # Edit display source ds_imp = a.Dataset2D() ds_imp.ImportFromFile(csvAmp) lineAmp.Update([ds_imp])
matplotlib chart
The idea is to extract the data from the two result objects, create the custom dataset, and plot directly in Mechanical using matplotlib. The script which can be executed with CPython:import csv import matplotlib.pyplot as plt ### Get Frequency Reponse object data ### respX = DataModel.GetObjectsByName('Frequency Response - X Deform')[0] respZ = DataModel.GetObjectsByName('Frequency Response - Z Deform')[0] tabDataX = respX.TabularData freqDataX = list(tabDataX['Frequency']) ampDataX = list(tabDataX['Amplitude']) phaseDataX = list(tabDataX['Phase Angle']) tabDataZ = respZ.TabularData freqDataZ = list(tabDataZ['Frequency']) ampDataZ = list(tabDataZ['Amplitude']) phaseDataZ = list(tabDataZ['Phase Angle']) freqData = freqDataX #Same frequency range for both results ampData = [aX / aZ for aX, aZ in zip(ampDataX, ampDataZ)] ### Plot using matplotlib ### plt.plot(freqData, ampData, marker = 'o', color = 'black', linestyle = '-') plt.xlim(min(freqData), max(freqData)) plt.ylim(min(ampData), max(ampData)) plt.xlabel('Frequency (Hz)') plt.ylabel('Amplitude (mm)') plt.show()
More info on the techniques above:
https://discuss.ansys.com/discussion/3114/how-to-export-frequency-response-amplitude-and-phase-from-mechanical
https://discuss.ansys.com/discussion/981/how-to-use-matplotlib-and-numpy-in-mechanical0