How can we automatically (say via mechanical scripting) create directional point masses?
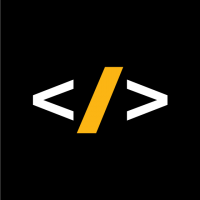
How can we automatically (say via mechanical scripting) create directional point masses, say by first reading some data from a csv file (where the first column in that file gives the named selection to scope to, and the other columns contain the directional mass in x, y , z respectively for that point mass - so every row contains data for a point mass)?
Best Answer
-
There are many ways of doing this. An attempt of such script is seen below.
It will read in the point mass data from the csv file , and based on this, create the point masses with their apdl command snippets that define the directional point mass data specified in the csv file.
import csv mx=[] my=[] mz=[] names=[] with open('D:\mymass.csv', 'rb') as f: reader = csv.reader(f) for row in reader: mz.append(row[3]) my.append(row[2]) mx.append(row[1]) names.append(row[0]) geometry = Model.Geometry for ii in range(0,len(names)): point_mass = geometry.AddPointMass() cs=point_mass.AddCommandSnippet() ns= DataModel.GetObjectsByName(str(names[ii]))[0] point_mass.Location=ns point_mass.Mass=Quantity('0.001 [kg]') cs.AppendText("keyopt,_tid,1,0\n") cs.AppendText("keyopt,_tid,3,0\n") cs.AppendText("r," +"_tid," +str(mx[ii]) + "," + str(my[ii]) +"," +str(mz[ii]) + "," + str(0) +"," + str(0) + "," + str(0) + "\n")
or in a single loop:
geometry = Model.Geometry with open('D:\mymass.csv', 'rb') as f: for line in f: mz=(line.Split(',')[3]).Split('\n')[0] my=(line.Split(',')[2]) mx=(line.Split(',')[1]) names=(line.Split(',')[0]) point_mass = geometry.AddPointMass() cs=point_mass.AddCommandSnippet() ns= DataModel.GetObjectsByName(str(names))[0] point_mass.Location=ns point_mass.Mass=Quantity('0.001 [kg]') cs.AppendText("keyopt,_tid,1,0\n") cs.AppendText("keyopt,_tid,3,0\n") cs.AppendText("r," +"_tid," +str(mx) + "," + str(my) +"," +str(mz) + "," + str(0) +"," + str(0) + "," + str(0) + "\n")
and something unrelated:
(retrieve selected object in the mechanical tree, and if point mass print yes)
ob=Tree.FirstActiveObject if (ob.GetType())==Ansys.ACT.Automation.Mechanical.PointMass: print('yes') else: print('no')
0