Using mech_dpf to output stress components in cylindrical coordinate systems
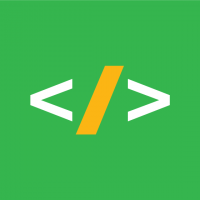
I want to output stress components for specific nodes, at specific time steps, of an analysis for further post processing.
I can do this in cartesian coordinate system but need the results in polar coordinate system.
I am currently working in 21R2, and would like to do this in mechanical scripting as I am not able to interact with mechanical from external python yet and the python results is still beta in 21R2.
Eventually I will export stresses to a file but for simplicity I am only printing now.
My questions are:
1) How can this be done without coding transformation calculations?
It seems feasible as shown here but I have not been able to implement this solution.
2) Can this be done by referencing and using existing polar coordinate system in the model?
3) Can I optimize requesting this data in the utilization of dpf for larger result files?
A simplified version of my current code is below:
import mech_dpf import Ans.DataProcessing as dpf # user inputs model_num = 0 node_num_list = [74] time_steps = [1,2,3,4,5,6,7,8,9,10] # get the analysis, result file, set the data source, and set the model analysis = Model.Analyses[model_num] rstFile=analysis.WorkingDir+"file.rst" data_source = dpf.DataSources(rstFile) model = dpf.Model(data_source) # set the location scoping nodal_scoping = dpf.Scoping(Location = dpf.locations.nodal) nodal_scoping.Ids = node_num_list # set the time scoping time_scoping = dpf.Scoping(Location = dpf.locations.time_freq_sets) time_scoping_list= [] for i in time_steps: # Note: the result time frequencies start at 0 ts = model.TimeFreqSupport.GetTimeFreqCummulativeIndex(i-1,-1)+1 time_scoping_list.append(ts) time_scoping.Ids = time_scoping_list # set requested values and the scoping opx = dpf.operators.result.stress_X() opy = dpf.operators.result.stress_Y() opz = dpf.operators.result.stress_Z() opxy = dpf.operators.result.stress_XY() opyz = dpf.operators.result.stress_YZ() opxz = dpf.operators.result.stress_XZ() operator_list = [opx, opy, opz, opxy, opyz, opxz] for op in operator_list: op.inputs.data_sources.Connect(data_source) op.inputs.mesh_scoping.Connect(nodal_scoping) op.inputs.time_scoping.Connect(time_scoping) # get the outputs output_list = [] for op in operator_list: output = op.outputs.fields_container.GetData() output_list.append(output) # print the history for i in time_steps: output_str = str(i) + ": " for output in output_list: output_val = output[i-1].Data[0] output_str += str(round(output_val,2)) + ", " print(output_str)