How to extract rotational displacements on a static analysis ?
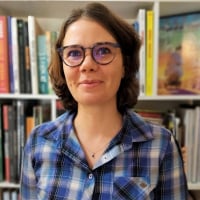
Pernelle Marone-Hitz
Member, Moderator, Employee Posts: 873
✭✭✭✭
How can I extract the rotational displacement values from the result file of a static structural analysis using PyDPF?
Tagged:
0
Answers
-
raw_displacement
operator can be used for that purpose. Please note that the data contained inraw_displacement
will depend on the types of elements used, the type of analysis, whether the model is 2D or 3D, etc.Below is an example:
- import ansys.dpf.core as dpf
- import numpy as np
- rstfile = r'D:/nodal_solution/file.rst'
- datfile = r'D:/nodal_solution/ds.dat'
- substep = 4
- # create the DataSource
- ds = dpf.DataSources()
- ds.set_result_file_path(rstfile, key="rst")
- ds.add_file_path(datfile, key="dat")
- # instantiate a Model
- model = dpf.Model(data_sources=ds)
- print(model)
- # define a time_scoping on substep 4
- time_scoping = dpf.time_freq_scoping_factory.scoping_by_step_and_substep_from_model(
- load_step_id=1,
- subset_id=substep,
- model=model
- )
- # extract the raw displacements
- displacement_fc = dpf.operators.result.raw_displacement(
- time_scoping=time_scoping,
- data_sources=model,
- ).eval()
- print(displacement_fc)
- # extract the field from the fields_container
- disp_field = displacement_fc[0]
- print(disp_field)
- # one can access the field's underlying numpy.ndarray using ".data"
- print(disp_field.data)
- # save to csv
- np.savetxt(
- 'displacement2_pydpf-core.csv',
- X=disp_field.data,
- delimiter=',', comments='', fmt='%.18e',
- header="UX, UY, UZ, RX, RY, RZ"
- )
- # optional: extract the rotational dofs from the field
- rotational_disp = dpf.operators.logic.component_selector(
- field=disp_field,
- component_number=[3, 4, 5]
- ).eval()
- print(rotational_disp)
0