Example of worflow automation: create geometry, model setup, and postprocess
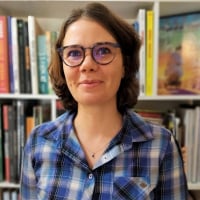
Is there an example of workflow automation with geometry creation, model setup and postprocessing?
Answers
-
Below is an example.
First, we'll create the geometry.
The script below will work both in SpaceClaim and in Discovery. It:
- creates a base block and four smaller blocks on the top
- defines a name for the base block
- creates four named selections (one for the base block body, one for the smaller blocks bodies, one for the bottom base face and one for the upper faces)
- # Python Script, API Version = V23
- ClearAll()
- # Geometry dimensions
- par_L = MM(50) # length of base block
- par_W = MM(25) # width of base block
- par_H = MM(10) # height of base block
- par_l = MM(20) # length of small block
- par_w = MM(10) # width of small block
- par_h = MM(5) # height of small block
- offset = MM(2.5)
- # Create base block
- base_block = BlockBody.Create(Point.Create(MM(0), MM(0), MM(0)), Point.Create(par_L, par_W, par_H ), ExtrudeType.ForceAdd)
- base_block.CreatedBodies[0].SetName('Base Block Body')
- my_body_selection = Selection.Create(base_block.CreatedBodies[0])
- my_body_selection.CreateAGroup('Base Block NS')
- my_faces_selection = my_body_selection.ConvertToFaces()
- for face in my_faces_selection.Items:
- if face.EvalMid().Point.Z == MM(0):
- Selection.Create(face).CreateAGroup('Base Face NS')
- # Create smaller blocks
- small_block_1 = BlockBody.Create(Point.Create(offset, offset, par_H ), Point.Create( par_L/2 - offset, par_w, par_H + par_h ), ExtrudeType.ForceIndependent)
- small_block_2 = BlockBody.Create(Point.Create(par_L/2 + offset, offset, par_H ), Point.Create(par_L-offset , par_w, par_H + par_h ), ExtrudeType.ForceIndependent)
- small_block_3 = BlockBody.Create(Point.Create(offset, par_W - offset-par_w, par_H ), Point.Create( par_L/2 - offset, par_W - offset , par_H + par_h ), ExtrudeType.ForceIndependent)
- small_block_4 = BlockBody.Create(Point.Create(par_L/2 + offset, par_W - offset-par_w, par_H ), Point.Create(par_L-offset , par_W - offset, par_H + par_h), ExtrudeType.ForceIndependent)
- my_body_selection = Selection.Create(small_block_1.CreatedBodies[0], small_block_2.CreatedBodies[0], small_block_3.CreatedBodies[0], small_block_4.CreatedBodies[0])
- my_body_selection.CreateAGroup('Small Blocks NS')
- my_faces_selection = my_body_selection.ConvertToFaces()
- top_face_list = []
- for face in my_faces_selection.Items:
- if face.EvalMid().Point.Z == par_H + par_h :
- top_face_list.append(face)
- Selection.Create(top_face_list).CreateAGroup('Top Faces NS')
In SpaceClaim, the resulting tree can be found in the "Structure" tab, and the named selections in the "Groups" tab:
In Discovery, the tree is on the top left:
and the named selections can be found in the "Advanced Selection" menu at the bottom right:
0 -
Then, we'll create the Mechanical model setup and postprocessing.
For the script to work, it is assumed that two materials have already been added in Engineering Data:
(these could also be created through scripting). It is also assumed that a Steady State thermal analysis is considered (the code could easily be adapted for other boundary conditions for other types of analyses).
The script will:
- add material assignements
- add boundary conditions based on named selections
- insert results
- export these results to a specified folder to extract screenshots of the plots, animations of the plots, and values of the plots
0 -
The Mechanical script is:
- # Retrieve bodies
- all_bodies = ExtAPI.DataModel.Project.Model.Geometry.GetChildren(DataModelObjectCategory.Body,True)
- base_block_body = [body for body in all_bodies if body.Name.Contains('Base')]
- small_block_bodies = [body for body in all_bodies if body.Name.Contains('Solid')]
- # Define material assignation
- material_assignation_steel = ExtAPI.DataModel.Project.Model.Materials.AddMaterialAssignment()
- sel = ExtAPI.SelectionManager.CreateSelectionInfo(SelectionTypeEnum.GeometryEntities)
- sel.Ids = [body.GetGeoBody().Id for body in base_block_body]
- material_assignation_steel.Location = sel
- material_assignation_steel.Material = 'Structural Steel'
- material_assignation_iron = ExtAPI.DataModel.Project.Model.Materials.AddMaterialAssignment()
- sel = ExtAPI.SelectionManager.CreateSelectionInfo(SelectionTypeEnum.GeometryEntities)
- sel.Ids = [body.GetGeoBody().Id for body in small_block_bodies]
- material_assignation_iron.Location = sel
- material_assignation_iron.Material = 'Aluminum'
- # Define boundary conditions based on Named Selections
- analysis = ExtAPI.DataModel.Project.Model.Analyses[0]
- temp_base = analysis.AddTemperature()
- temp_base.Location = ExtAPI.DataModel.GetObjectsByName("Base Face NS")[0]
- temp_base.Magnitude.Output.SetDiscreteValue(0, Quantity(50, "C"))
- convection = analysis.AddConvection()
- convection.Location = ExtAPI.DataModel.GetObjectsByName("Top Faces NS")[0]
- convection.FilmCoefficient.Output.SetDiscreteValue(0, Quantity(25, "W m^-1 m^-1 C^-1"))
- convection.AmbientTemperature.Output.SetDiscreteValue(0, Quantity(5, "C"))
- # Solve model
- analysis.Solve()
- # Insert results
- solution = ExtAPI.DataModel.Project.Model.Analyses[0].Solution
- temp_plot = solution.AddTemperature()
- temp_plot_on_ns = solution.AddTemperature()
- temp_plot_on_ns.Location = ExtAPI.DataModel.GetObjectsByName("Top Faces NS")[0]
- solution.EvaluateAllResults()
- # Export results
- import os
- export_folder = r'D:\Data'
- all_results = solution.GetChildren(DataModelObjectCategory.Result,True)
- for result in all_results:
- export_name = os.path.join(export_folder,result.Name)
- ExtAPI.Graphics.ExportImage(export_name +'.png')
- result.ExportAnimation(export_name + '.avi')
- result.ExportToTextFile(export_name + '.txt')
0