Plot field Vectors on a Named Selection in PyDPF
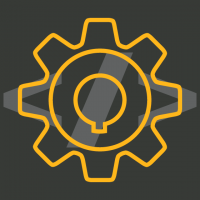
George Tzortzopoulos
Member, Employee Posts: 12
✭✭✭
Comments
-
For generating a vector plot on a named selection in PyDPF, some functions of the PyVista Python library are needed. The example presented below is a combination of the PyDPF and PyVista open-source codes. Here is an example of working with the Heat Flux field:
- from ansys.dpf import core as dpf
- from ansys.dpf.core.plotter import DpfPlotter
- from ansys.dpf.core.common import locations
- import pyvista as pv
- import numpy as np
- """
- Load result file and create model database
- """
- path = "my_Path"
- ds = dpf.DataSources(path)
- model = dpf.Model(ds)
- mesh = model.metadata.meshed_region
- """
- Select preferable Named Selection
- """
- get_NSs = model.metadata.available_named_selections
- my_mesh_scoping = model.metadata.named_selection("my_Named_Selection")
- """
- Scope Named Selection to corresponding Mesh
- """
- scoping_op = dpf.operators.mesh.from_scoping()
- scoping_op.inputs.scoping.connect(my_mesh_scoping)
- scoping_op.inputs.mesh.connect(mesh)
- my_mesh = scoping_op.outputs.mesh()
- """
- Get Heat Flux results at last time step
- """
- get_all_heatFlux = model.results.heat_flux.on_all_time_freqs
- get_fieldContents_heatFlux = get_all_heatFlux(mesh_scoping=my_mesh_scoping).eval()
- get_field_heatFlux = get_fieldContents_heatFlux[-1]
- """
- Recreate DpfPlotter() and Contour Plot based on PyDPF Source Code and PyVista library
- """
- sargs = dict(
- title = "Heat Flux [W/m^2]",
- title_font_size = 30,
- label_font_size = 20,
- interactive = True
- )
- margs = dict(
- show_edges = True,
- nan_color = None,
- )
- field = get_field_heatFlux
- meshed_region = my_mesh
- location = field.location
- show_max = False
- show_min = False
- mesh_location = meshed_region.nodes
- component_count = field.component_count
- overall_data = np.full((len(mesh_location), component_count), np.nan)
- ind, mask = mesh_location.map_scoping(field.scoping)
- overall_data[ind] = field.data[mask]
- deform_by = None
- scale_factor=1.0
- as_linear=True
- grid = meshed_region.grid
- grid.set_active_scalars(None)
- p = pv.Plotter()
- p.add_mesh(mesh=grid, scalars=overall_data, **margs, scalar_bar_args=sargs)
- p.add_axes()
- p.show()
- """
- Vector Plot
- """
- scaling = 1e-7
- grid["my_vectors"] = overall_data * scaling
- grid.set_active_vectors("my_vectors")
- vtitle = "Vector Magnitude"
- vargs = dict(title = vtitle)
- p = pv.Plotter()
- p.add_mesh(grid.arrows, lighting=False, scalar_bar_args=vargs)
- p.add_mesh(grid, scalars=overall_data, **margs, scalar_bar_args=sargs)
- p.remove_scalar_bar(title=vtitle)
- p.add_axes()
- p.show()
0