How to calculate contact surface and heat flow with the use of PyDpf
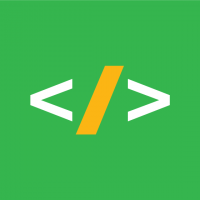
Kleanthis
Member, Employee Posts: 6
✭✭✭
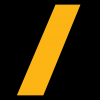
How to calculate contact surface and heat flow with the use of PyDpf
Tagged:
0
Comments
-
The script below captures the contact for each element by getting the CONTAC174 element and calculates its contact surface. Afterwards it calculates the heat flux of each element.
A prerequisite for the generation of the contact surface named selection is to add the following APDL command under the desired contact:
- my_cont=cid
And the following command object before solution:
- /PREP7
- ESEL, S, TYPE, , my_cont
- CM, PCB_CONT, ELEM
- ALLSEL
- /SOLU
The PyDPF script is as follows:
- from ansys.dpf import core as dpf
- from ansys.dpf import core as dpf
- from ansys.dpf.core.plotter import DpfPlotter
- import numpy as np
- """
- Load result file and create model database
- """
- ds = dpf.DataSources("file.rth")
- model = dpf.Model(ds)
- # %%
- get_NSs = model.metadata.available_named_selections
- print(get_NSs)
- # %%
- mesh_scoping = model.metadata.named_selection("PCB_CONT_2")
- print(mesh_scoping)
- # %%
- print(get_NSs)
- mesh_scoping = model.metadata.named_selection("PCB_CONT_2")
- print(mesh_scoping)
- # %%
- contact_status_op = dpf.operators.result.nmisc(
- # time_scoping=-1,
- mesh_scoping=mesh_scoping,
- # fields_container=my_fields_container,
- # streams_container=my_streams_container,
- data_sources=ds,
- # mesh=my_mesh,
- item_index=41,
- # num_components=my_num_components,
- )
- print(contact_status_op)
- # %%
- contact_status_res = contact_status_op.outputs.fields_container()
- print(contact_status_res)
- # %%
- scoping_op = dpf.operators.mesh.from_scoping()
- scoping_op.inputs.scoping.connect(mesh_scoping)
- scoping_op.inputs.mesh.connect(contact_status_res[0].meshed_region)
- my_mesh = scoping_op.outputs.mesh()
- print(my_mesh)
- # %%
- plot = DpfPlotter()
- plot.add_field(contact_status_res[0], my_mesh, notebook=False)
- plot.show_figure(show_axes=True)
- # %%
- touch_cond = (contact_status_res[0].data >= 2.)
- Nelems_touch = sum(touch_cond)
- print("Number of Touching Elements: ", Nelems_touch)
- print("Number of Total Elements: ", len(contact_status_res[0].data))
- # %%
- contact_area_op = dpf.operators.result.nmisc(
- # time_scoping=-1,
- mesh_scoping=mesh_scoping,
- # fields_container=my_fields_container,
- # streams_container=my_streams_container,
- data_sources=ds,
- # mesh=my_mesh,
- item_index=58,
- # num_components=my_num_components,
- )
- print(contact_area_op)
- # %%
- contact_area_res = contact_area_op.outputs.fields_container()
- print(contact_area_res)
- # %%
- print(contact_area_res[0].data)
- # %%
- scoping_op = dpf.operators.mesh.from_scoping()
- scoping_op.inputs.scoping.connect(mesh_scoping)
- scoping_op.inputs.mesh.connect(contact_area_res[0].meshed_region)
- my_mesh = scoping_op.outputs.mesh()
- print(my_mesh)
- # %%
- plot = DpfPlotter()
- plot.add_field(contact_area_res[0], my_mesh, show_max=True, show_min=True, notebook=False)
- plot.show_figure(show_axes=True)
- # %%
- contact_heatFlux_op = dpf.operators.result.smisc(
- # time_scoping=-1,
- mesh_scoping=mesh_scoping,
- # fields_container=my_fields_container,
- # streams_container=my_streams_container,
- data_sources=ds,
- # mesh=my_mesh,
- item_index=14,
- # num_components=my_num_components,
- )
- print(contact_heatFlux_op)
- # %%
- contact_heatFlux_res = contact_heatFlux_op.outputs.fields_container()
- print(contact_heatFlux_res)
- # %%
- scoping_op = dpf.operators.mesh.from_scoping()
- scoping_op.inputs.scoping.connect(mesh_scoping)
- scoping_op.inputs.mesh.connect(contact_heatFlux_res[0].meshed_region)
- my_mesh = scoping_op.outputs.mesh()
- print(my_mesh)
- # %%
- plot = DpfPlotter()
- plot.add_field(contact_heatFlux_res[0], my_mesh, show_max=True, show_min=True, notebook=False)
- plot.show_figure(show_axes=True)
1