How can I perform a parametric analysis through PyMechanical?
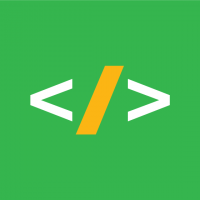
MSensale
Member, Employee Posts: 4
✭✭✭
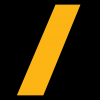
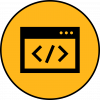
Here is an example code to perform a parametric analysis through PyMechanical with parameters that are not parametrized by Mechanical itself.
Tagged:
2
Comments
-
Here is the code :
- from ansys.mechanical.core import launch_mechanical
- from pathlib import Path
- ###############################################################################
- # Launch Mechanical
- # ~~~~~~~~~~~~~~~~~
- # Launch a new Mechanical session in batch, setting ``cleanup_on_exit`` to
- # ``False``. To close this Mechanical session when finished, this example
- # must call the ``mechanical.exit()`` method.
- mechanical = launch_mechanical(batch=False, cleanup_on_exit=False)
- print(mechanical)
- ###############################################################################
- # Define parameters
- # ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
- P1 = [0.001, 0.005, 0.01, 0.05, 0.1] # Maximum Time Step
- P2 = [3, 2.5, 2, 1.5, 1, 0.5, 0.25] # Face Sizing and Face Sizing 2
- P3 = ["Linear"] #Element order
- P4 = ["PurePenalty"] #Contact formulation
- P5 = ["NodalNormalToTarget", "NodalProjectedNormalFromContact", "NodalDualShapeFunctionProjection"] # Detection Method
- names = ['Max Time Step', 'Sizing', 'Order', 'Formulation', 'Detection']
- parameters = [P1,P2,P3,P4,P5]
- param = dict(zip(names, parameters))
- for key, value in param.items():
- length = (len(value))
- for j in range(0, length):
- print(value[j])
- ###################################################################################
- # Open the Mechanical model
- # ~~~~~~~~~~~~~~~~~~
- # Run the Mechanical script to attach the geometry and set up and solve the
- # analysis.
- file = r"D:\\Data\\project1\\SYS-99.mechdb"
- command = f'ExtAPI.DataModel.Project.Open("{file}")'
- # RUN LINES TO PERFORM PRELIMINARY OPERATIONS
- # ~~~~~~~~~~~~~~~~~~
- mechanical.run_python_script(command)
- command = """
- import json
- #Scenario 1: Store main Tree Object items
- MODEL = Model
- GEOM = MODEL.Geometry
- COORDINATE_SYSTEMS = Model.CoordinateSystems
- MESH = Model.Mesh
- NAMED_SELECTIONS = Model.NamedSelections
- CONNECTIONS = Model.Connections
- PARTS = GEOM.GetChildren(DataModelObjectCategory.Part,False)
- for part in PARTS:
- bodies = part.GetChildren(DataModelObjectCategory.Body, False)
- for body in bodies:
- if body.Name == "Part 1":
- PART1 = body
- STAT_STRUC = DataModel.Project.Model.Analyses[0]
- ANALYSIS_SETTINGS = STAT_STRUC.AnalysisSettings
- STAT_STRUC_SOLUTION = STAT_STRUC.Solution"""
- output = mechanical.run_python_script(command)
- print(output)
- # CHANGE THE PARAMETER
- # ~~~~~~~~~~~~~~~~~~
- if key == 'Max Time Step':
- command = """
- ANALYSIS_SETTINGS.Activate()
- ANALYSIS_SETTINGS.CurrentStepNumber=2
- ANALYSIS_SETTINGS.MaximumTimeStep=Quantity("{0:.3f} [sec]")
- """.format(value[j])
- if key == 'Sizing':
- command = """
- MESH.Children[1].ElementSize = Quantity({0:.1f}, "m")
- MESH.Children[2].ElementSize = Quantity({0:.1f}, "m")""".format(value[j])
- if key == 'Order':
- command = """
- MESH.ElementOrder = ElementOrder.{0:s}""".format(value[j])
- if key == 'Formulation':
- command = """
- CONNECTIONS.Children[0].Children[0].ContactFormulation = ContactFormulation.{0:s}
- CONNECTIONS.Children[0].Children[1].ContactFormulation = ContactFormulation.{0:s}""".format(value[j])
- if key == 'Detection':
- command = """
- CONNECTIONS.Children[0].Children[0].DetectionMethod = ContactDetectionPoint.{0:s}
- CONNECTIONS.Children[0].Children[1].DetectionMethod = ContactDetectionPoint.{0:s}""".format(value[j])
- print(command)
- output = mechanical.run_python_script(command)
- print(output)
- # UPDATE MESH AND SOLVE
- # ~~~~~~~~~~~~~~~~~~
- command = """
- # Solve Static Analysis
- MESH.GenerateMesh()
- STAT_STRUC = Model.Analyses[0]
- STAT_STRUC.Solution.Solve(True)"""
- output = mechanical.run_python_script(command)
- print(output)
2